Java Reference
In-Depth Information
36
public void remove( )
37
{
38
if( expectedModCount != modCount )
39
throw new ConcurrentModificationException( );
40
if( lastVisited == null )
41
throw new IllegalStateException( );
42
43
LinkedList.this.remove( lastVisited );
44
lastVisited = null;
45
if( lastMoveWasPrev )
46
current = current.next;
47
expectedModCount++;
48
}
49
50
public boolean hasPrevious( )
51
{
52
if( expectedModCount != modCount )
53
throw new ConcurrentModificationException( );
54
return current != beginMarker.next;
55
}
56
57
public AnyType previous( )
58
{
59
if( !hasPrevious( ) )
60
throw new NoSuchElementException( );
61
62
current = current.prev;
63
lastVisited = current;
64
lastMoveWasPrev = true;
65
return current.data;
66
}
67
}
figure 17.30b
Iterator inner class
implementation for
standard
LinkedList
class (
continued
)
remove
. If
lastVisited
is
null
, the
remove
is illegal. Finally,
lastMoveWasPrev
is
true
if the last movement of the iterator prior to
remove
was via
previous
; it is
false
if the last movement was via
next
.
The
hasNext
and
hasPrevious
methods are fairly routine. Both throw an
exception if an external modification to the list has been detected.
The
next
method advances
current
(line 32) after getting the value in the
node (line 30) that is to be returned (line 34). Data fields
lastVisited
and
lastMoveWasPrev
are updated at lines 31 and 33, respectively. The implemen-
tation of
previous
is not exactly symmetric, because for
previous
, we
advance
current
prior to obtaining the value. This is evident when one con-
siders that the initial state for backwards iteration is that
current
is at the
endmarker.
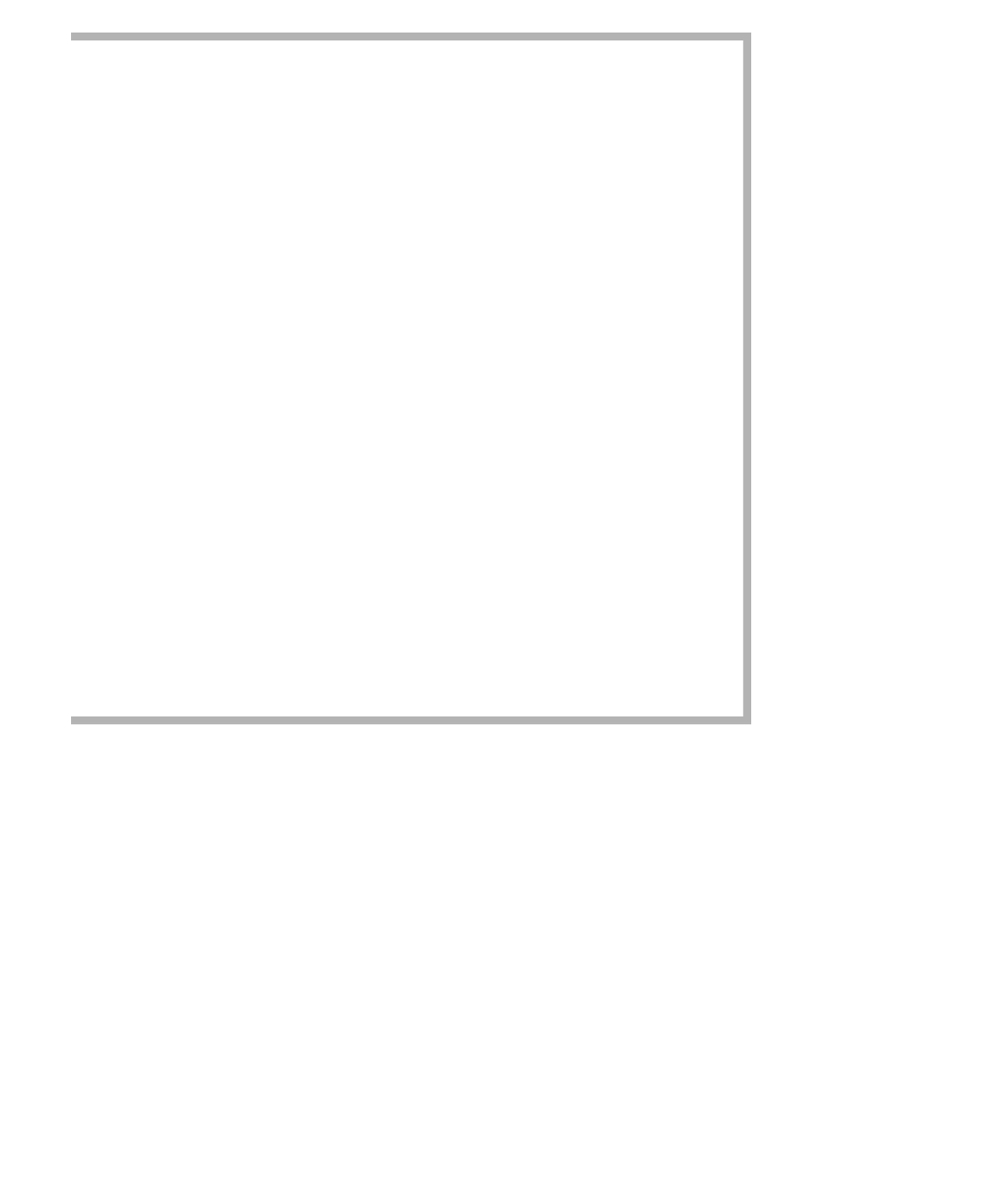
Search WWH ::

Custom Search