Java Reference
In-Depth Information
figure 9.5
Generation of a
random number
according to the
Poisson distribution
1
/**
2
* Return an int using a Poisson distribution, and
3
* change the internal state.
4
* @param expectedValue the mean of the distribution.
5
* @return the pseudorandom int.
6
*/
7
public int nextPoisson( double expectedValue )
8
{
9
double limit = -expectedValue;
10
double product = Math.log( nextDouble( ) );
11
int count;
12
13
for( count = 0; product > limit; count++ )
14
product += Math.log( nextDouble( ) );
15
16
return count;
17
}
Another important nonuniform distribution is the
negative exponential
distribution,
shown in Figure 9.6, which has the same mean and variance
and is used to model the time between occurrences of random events. We
use it in the simulation application shown in Section 13.2.
Many other distributions are commonly used. Our main purpose here is to
show that most can be generated from the uniform distribution. Consult any
book on probability and statistics to find out more about these functions.
The
negative expo-
nential distribution
has the same mean
and variance. It is
used to model the
time between
occurrences of ran-
dom events.
generating a random
permutation
9.4
Consider the problem of simulating a card game. The deck consists of 52
distinct cards, and in the course of a deal, we must generate cards from the deck,
without duplicates. In effect, we need to shuffle the cards and then iterate
figure 9.6
Generation of a
random number
according to the
negative exponential
distribution
1
/**
2
* Return a double using a negative exponential
3
* distribution, and change the internal state.
4
* @param expectedValue the mean of the distribution.
5
* @return the pseudorandom double.
6
*/
7
public double nextNegExp( double expectedValue )
8
{
9
return - expectedValue * Math.log( nextDouble( ) );
10
}
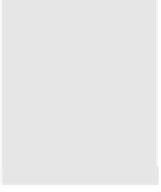


Search WWH ::

Custom Search