Java Reference
In-Depth Information
A recursive algorithm requires specifying a base case. When the size of
the problem reaches one element, we do not use recursion. The resulting Java
method is coded in Figure 7.20.
figure 7.20
A divide-and-conquer
algorithm for the
maximum contiguous
subsequence sum
problem
1
/**
2
* Recursive maximum contiguous subsequence sum algorithm.
3
* Finds maximum sum in subarray spanning a[left..right].
4
* Does not attempt to maintain actual best sequence.
5
*/
6
private static int maxSumRec( int [ ] a, int left, int right )
7
{
8
int maxLeftBorderSum = 0, maxRightBorderSum = 0;
9
int leftBorderSum = 0, rightBorderSum = 0;
10
int center = ( left + right ) / 2;
11
12
if( left == right ) // Base case
13
return a[ left ] > 0 ? a[ left ] : 0;
14
15
int maxLeftSum = maxSumRec( a, left, center );
16
int maxRightSum = maxSumRec( a, center + 1, right );
17
18
for( int i = center; i >= left; i-- )
19
{
20
leftBorderSum += a[ i ];
21
if( leftBorderSum > maxLeftBorderSum )
22
maxLeftBorderSum = leftBorderSum;
23
}
24
25
for( int i = center + 1; i <= right; i++ )
26
{
27
rightBorderSum += a[ i ];
28
if( rightBorderSum > maxRightBorderSum )
29
maxRightBorderSum = rightBorderSum;
30
}
31
32
return max3( maxLeftSum, maxRightSum,
33
maxLeftBorderSum + maxRightBorderSum );
34
}
35
36
/**
37
* Driver for divide-and-conquer maximum contiguous
38
* subsequence sum algorithm.
39
*/
40
public static int maxSubsequenceSum( int [ ] a )
41
{
42
return a.length > 0 ? maxSumRec( a, 0, a.length - 1 ) : 0;
43
}
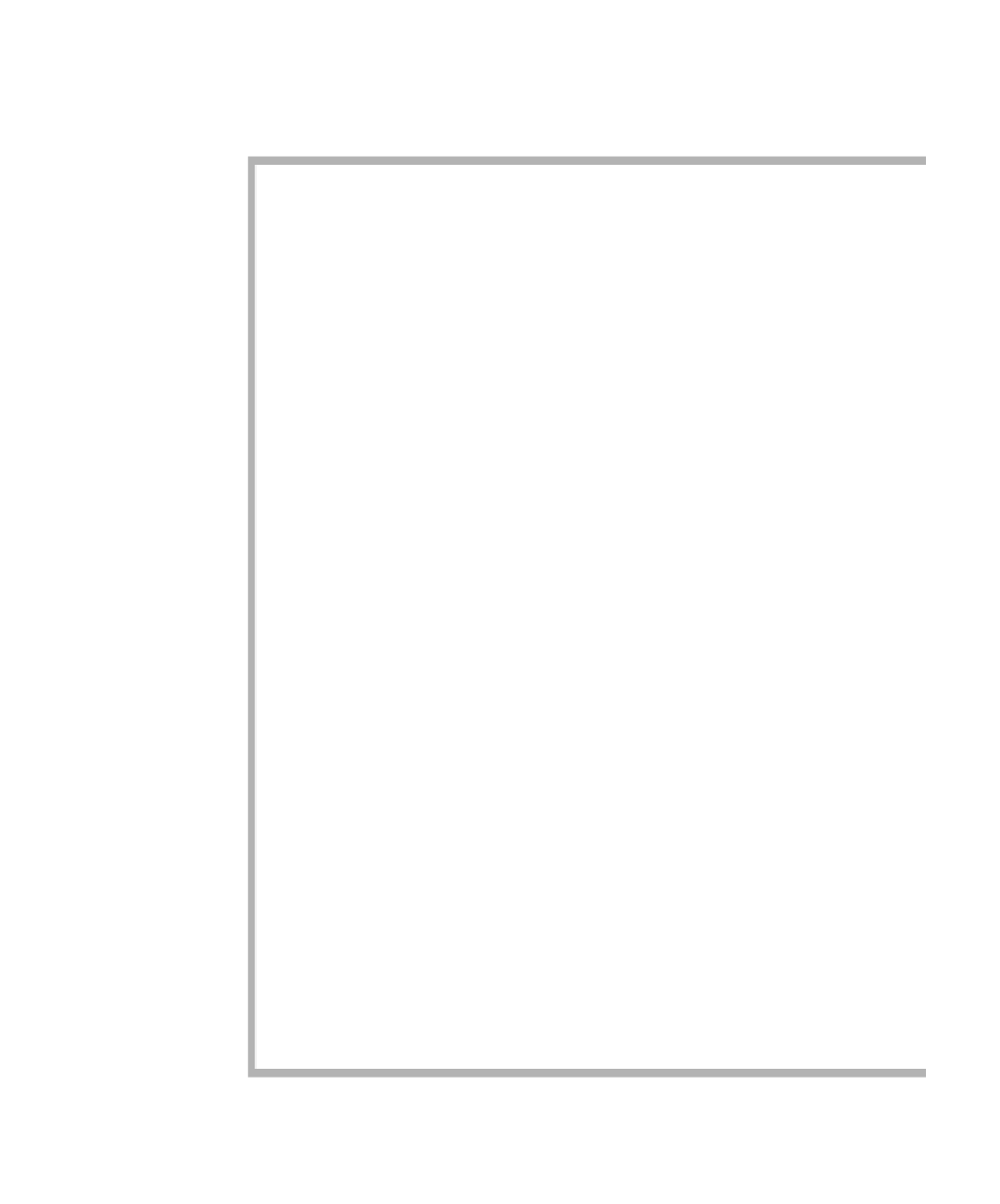
Search WWH ::

Custom Search