Java Reference
In-Depth Information
6.4.1
Comparator
function objects
Many Collections API classes and routines require the ability to order objects.
There are two ways to do this. One possibility is that the objects implement the
Comparable
interface and provide a
compareTo
method. The other possibility is
that the comparison function is embedded as the
compare
method in an object that
implements the
Comparator
interface.
Comparator
is defined in
java.util
; a sam-
ple implementation was shown in Figure 4.39 and is repeated in Figure 6.12.
6.4.2
the
Collections
class
Although we will not make use of the
Collections
class in this text, it has two
methods that are thematic of how generic algorithms for the Collections API
are written. We write these methods in the
Collections
class implementation
that spans Figures 6.13 and 6.14.
Figure 6.13 begins by illustrating the common technique of declaring a
private constructor in classes that contain only static methods. This prevents
instantiation of the class. It continues by providing the
reverseOrder
method.
This is a factory method that returns a
Comparator
that provides the reverse
of the natural ordering for
Comparable
objects. The returned object, created at
line 20, is an instance of the
ReverseComparator
class written in lines 23 to
29. In the
ReverseComparator
class, we use the
compareTo
method. This is an
reverseOrder
is a
factory method
that creates a
Comparator
repre-
senting the
reverse natural
order.
1
package weiss.util;
2
3
/**
4
* Comparator function object interface.
5
*/
6
public interface Comparator<AnyType>
7
{
8
/**
9
* Return the result of comparing lhs and rhs.
10
* @param lhs first object.
11
* @param rhs second object.
12
* @return < 0 if lhs is less than rhs,
13
* 0 if lhs is equal to rhs,
14
* > 0 if lhs is greater than rhs.
15
* @throws ClassCastException if objects cannot be compared.
16
*/
17
int compare( AnyType lhs, AnyType rhs ) throws ClassCastException;
18
}
figure 6.12
The
Comparator
interface, originally defined in
java.util
and rewritten for the
weiss.util
package
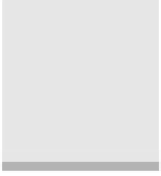
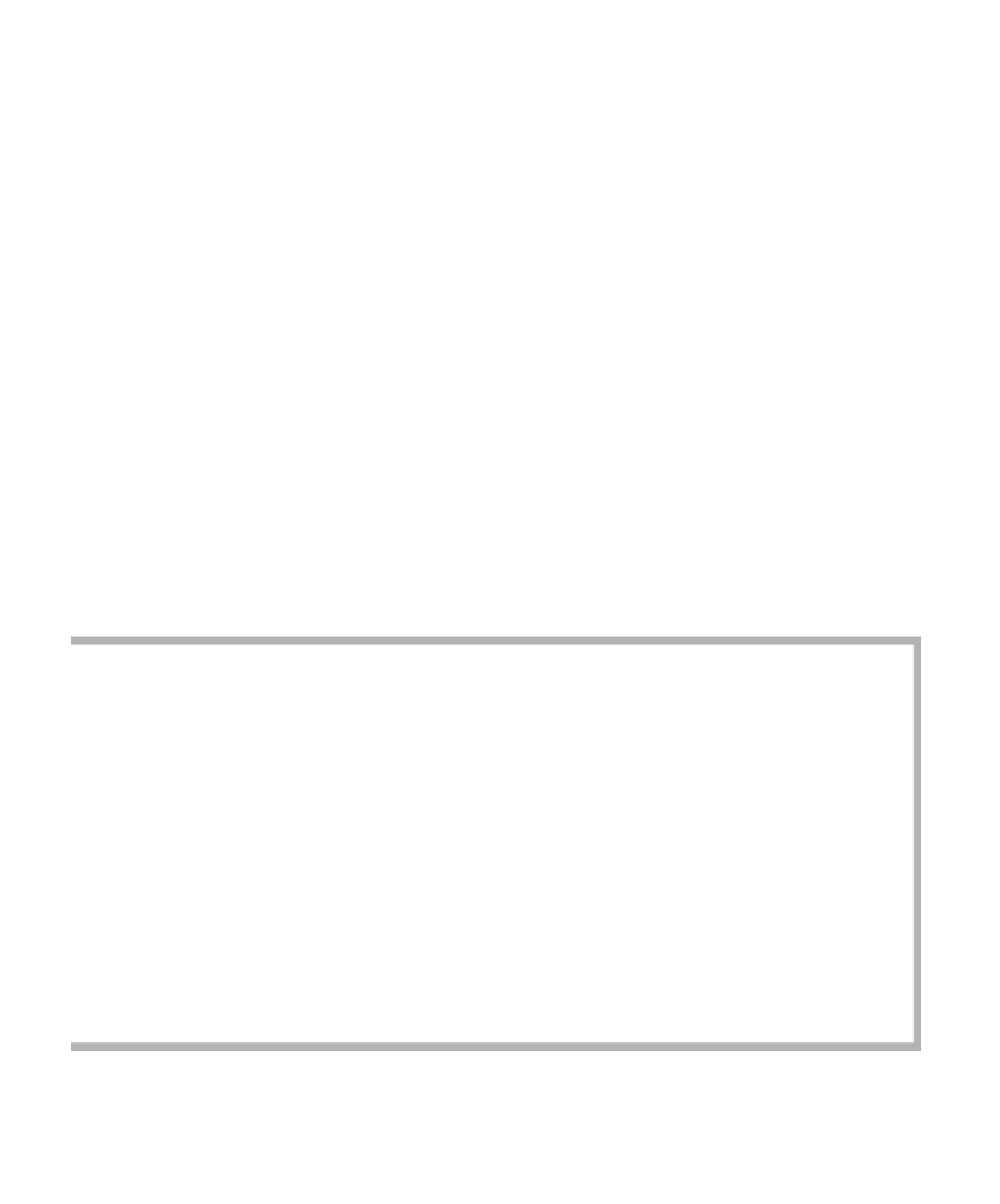
Search WWH ::

Custom Search