Java Reference
In-Depth Information
API takes a strict view: Any external structural modification of the container
(adds, removes, and so on) will result in a
ConcurrentModificationException
by
the iterator methods when one of the methods is called. In other words, if we
have an iterator, and then an object is added to the container, and then we invoke
the
next
method on the iterator, the iterator will detect that it is now invalid, and
an exception will be thrown by
next
.
This means that it is impossible to remove an object from a container
when we have seen it via an iterator, without invalidating the iterator. This is
one reason why there is a
remove
method in the iterator class. Calling the itera-
tor
remove
causes the last seen object to be removed from the container. It
invalidates all other iterators that are viewing this container, but not the itera-
tor that performed the
remove
. It is also likely to be more efficient than the con-
tainer's
remove
method, at least for some collections. However,
remove
cannot
be called twice in a row. Furthermore,
remove
preserves the semantics of
next
and
hasNext
, because the next unseen item in the iteration remains the same.
This version of
remove
is listed as an optional method, so the programmer
needs to check that it is implemented. The design of
remove
has been criticized
as poor, but we will use it at one point in the text.
Figure 6.10 provides a sample specification of the
Iterator
interface.
(Our iterator class extends the standard
java.util
version in order to allow
The
Iterator
methods throw an
exception if its
container has
been structurally
modified.
1
package weiss.util;
2
3
/**
4
* Iterator interface.
5
*/
6
public interface Iterator<AnyType> extends java.util.Iterator<AnyType>
7
{
8
/**
9
* Tests if there are items not yet iterated over.
10
*/
11
boolean hasNext( );
12
13
/**
14
* Obtains the next (as yet unseen) item in the collection.
15
*/
16
AnyType next( );
17
18
/**
19
* Remove the last item returned by next.
20
* Can only be called once after next.
21
*/
22
void remove( );
23
}
figure 6.10
A sample specification of
Iterator
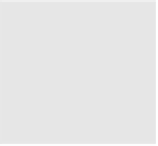





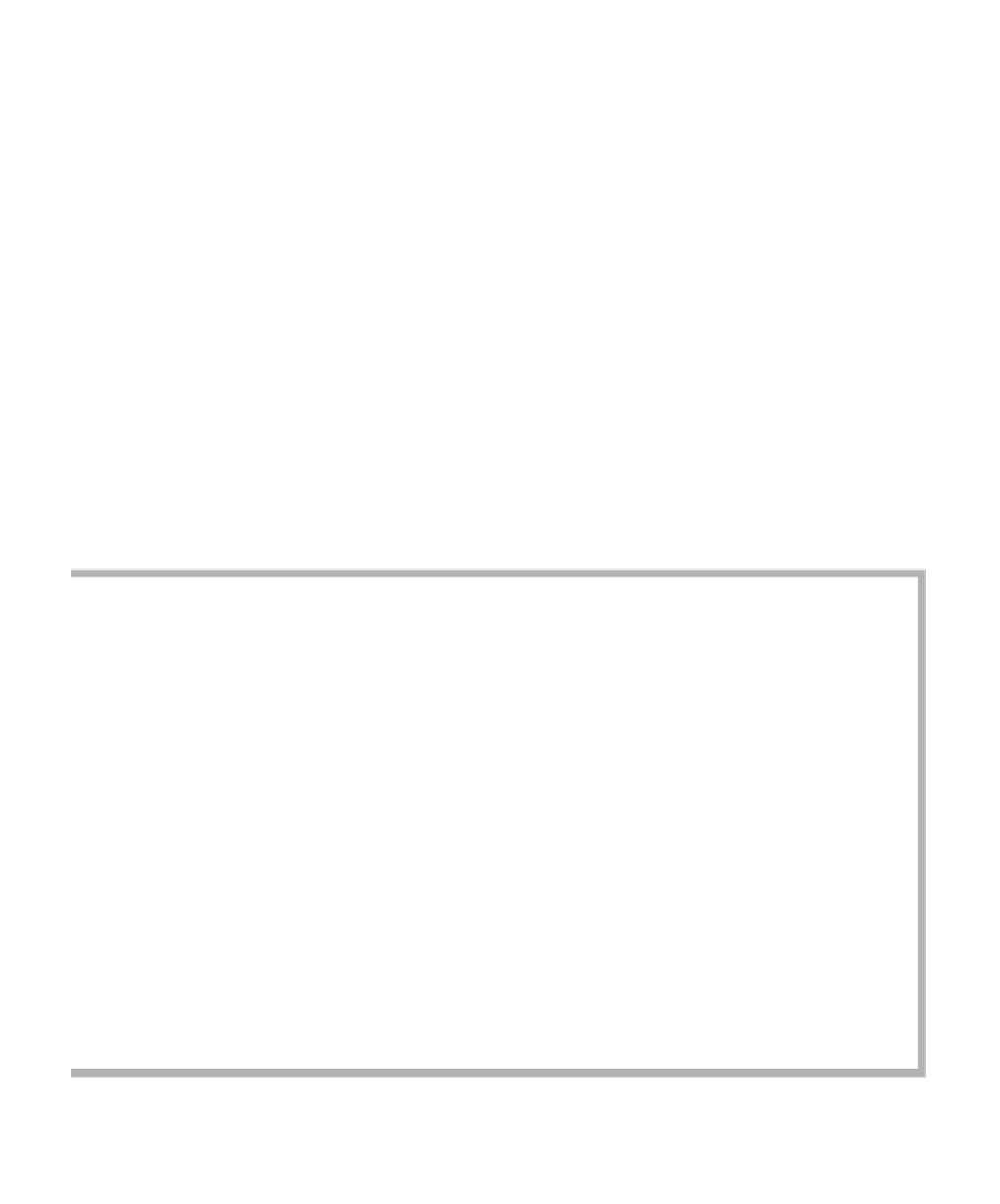
Search WWH ::

Custom Search