Java Reference
In-Depth Information
figure 4.13
A sample
program that
uses the
shape
hierarchy
1
class ShapeDemo
2
{
3
public static double totalArea( Shape [ ] arr )
4
{
5
double total = 0;
6
7
for( Shape s : arr )
8
if( s != null )
9
total += s.area( );
10
11
return total;
12
}
13
14
public static void printAll( Shape [ ] arr )
15
{
16
for( Shape s : arr )
17
System.out.println( s );
18
}
19
20
public static void main( String [ ] args )
21
{
22
Shape [ ] a = { new Circle( 2.0 ), new Rectangle( 1.0, 3.0 ), null };
23
24
System.out.println( "Total area = " + totalArea( a ) );
25
printAll( a );
26
}
27
}
A better solution for
area
is to throw a runtime exception (a good one is
UnsupportedOperationException
) in the
Shape
class. This is preferable to return-
ing -1 because the exception will not be ignored.
However, even that solution resolves the problem at runtime. It would be
better to have syntax that explicitly states that
area
is a placeholder and does
not need any implementation at all, and that further,
Shape
is a placeholder
class and cannot be constructed, even though it may declare constructors, and
will have a default constructor if none are declared. If this syntax were available,
then the compiler could, at compile time, declare as illegal any attempts to
construct a
Shape
instance. It could also declare as illegal any classes, such as
Triangle
, for which there are attempts to construct instances, even though
area
has not been overridden. This exactly describes abstract methods and
abstract classes.
Abstract methods
and classes repre-
sent placeholders.
An
abstract method
is a method that declares functionality that all derived
class objects must eventually implement. In other words, it says what these
objects can do. However, it does not provide a default implementation.
Instead, each object must provide its own implementation.
An
abstract method
has no meaningful
definition and is
thus always defined
in the derived class.
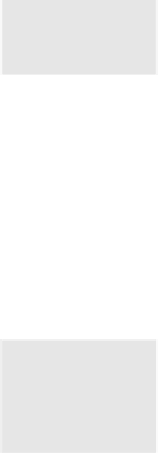




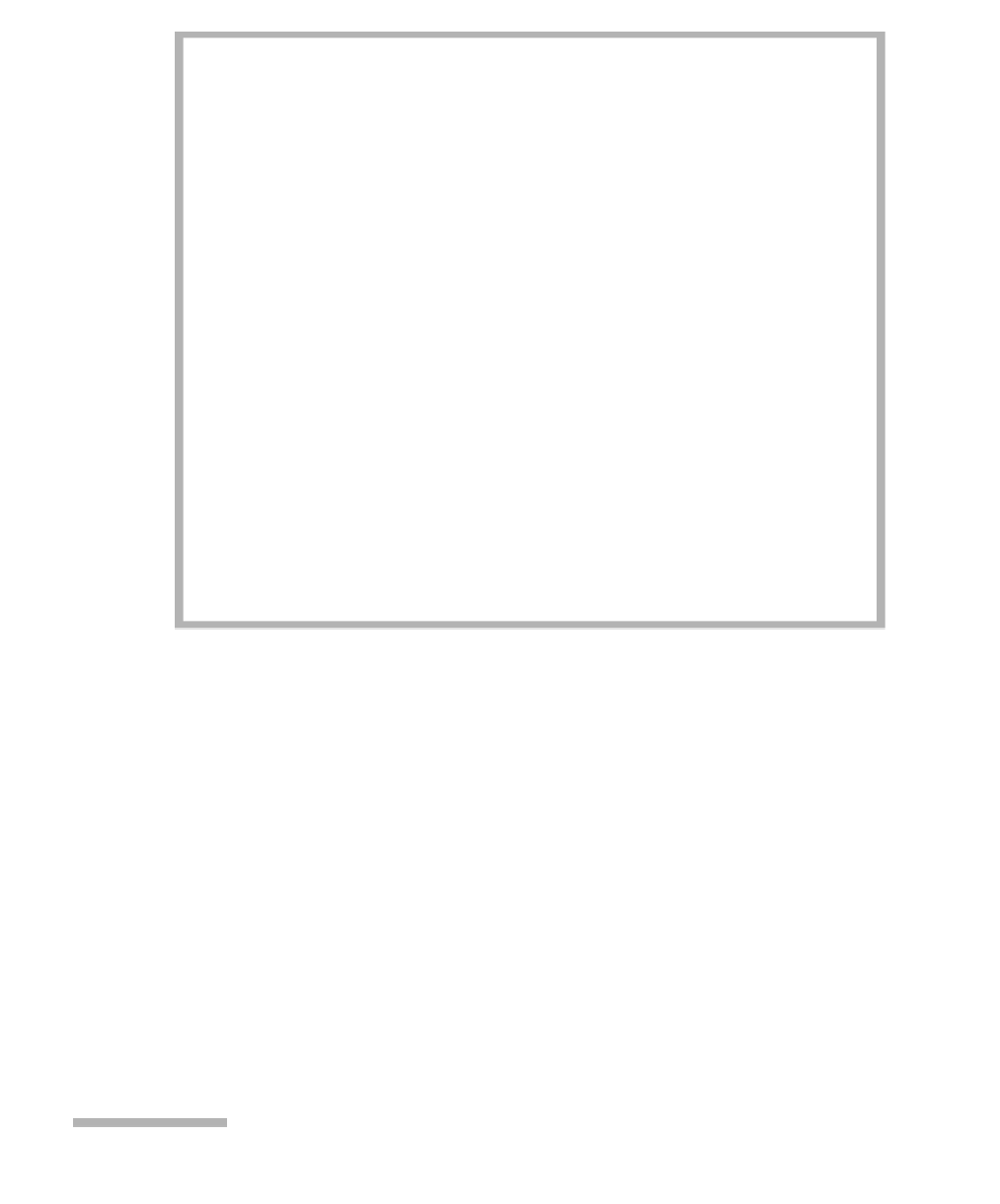
Search WWH ::

Custom Search