Java Reference
In-Depth Information
figure 4.9
An illustration of
polymorphism with
arrays
1
class PersonDemo
2
{
3
public static void printAll( Person [ ] arr )
4
{
5
for( int i = 0; i < arr.length; i++ )
6
{
7
if( arr[ i ] != null )
8
{
9
System.out.print( "[" + i + "] " );
10
System.out.println( arr[ i ].toString( ) );
11
}
12
}
13
}
14
15
public static void main( String [ ] args )
16
{
17
Person [ ] p = new Person[ 4 ];
18
19
p[0] = new Person( "joe", 25, "New York",
20
"212-555-1212" );
21
p[1] = new Student( "jill", 27, "Chicago",
22
"312-555-1212", 4.0 );
23
p[3] = new Employee( "bob", 29, "Boston",
24
"617-555-1212", 100000.0 );
25
26
printAll( p );
27
}
28
}
different hierarchy), the compiler will complain. If it is possible for the cast to
make sense, the program will compile, and so the above code will success-
fully give a 4% raise to
p[3]
. This construct, in which we change the static
type of an expression from a base class to a class farther down in the inheri-
tance hierarchy is known as a
downcast
.
What if
p[3]
was not an
Employee
? For instance, what if we used the following?
A
downcast
is a
cast down the
inheritance hierar-
chy. Casts are
always verified at
runtime by the
Virtual Machine.
((Employee) p[1]).raise( 0.04 ); // p[1] is a Student
In that case the program would compile, but the Virtual Machine would throw
a
ClassCastException
, which is a run-time exception that signals a program-
ming error. Casts are always double-checked at run time to ensure that the
programmer (or a malicious hacker) is not trying to subvert Java's strong
typing system. The safe way of doing these types of calls is to use
instanceof
first:
if( p[3] instanceof Employee )
((Employee) p[3]).raise( 0.04 );
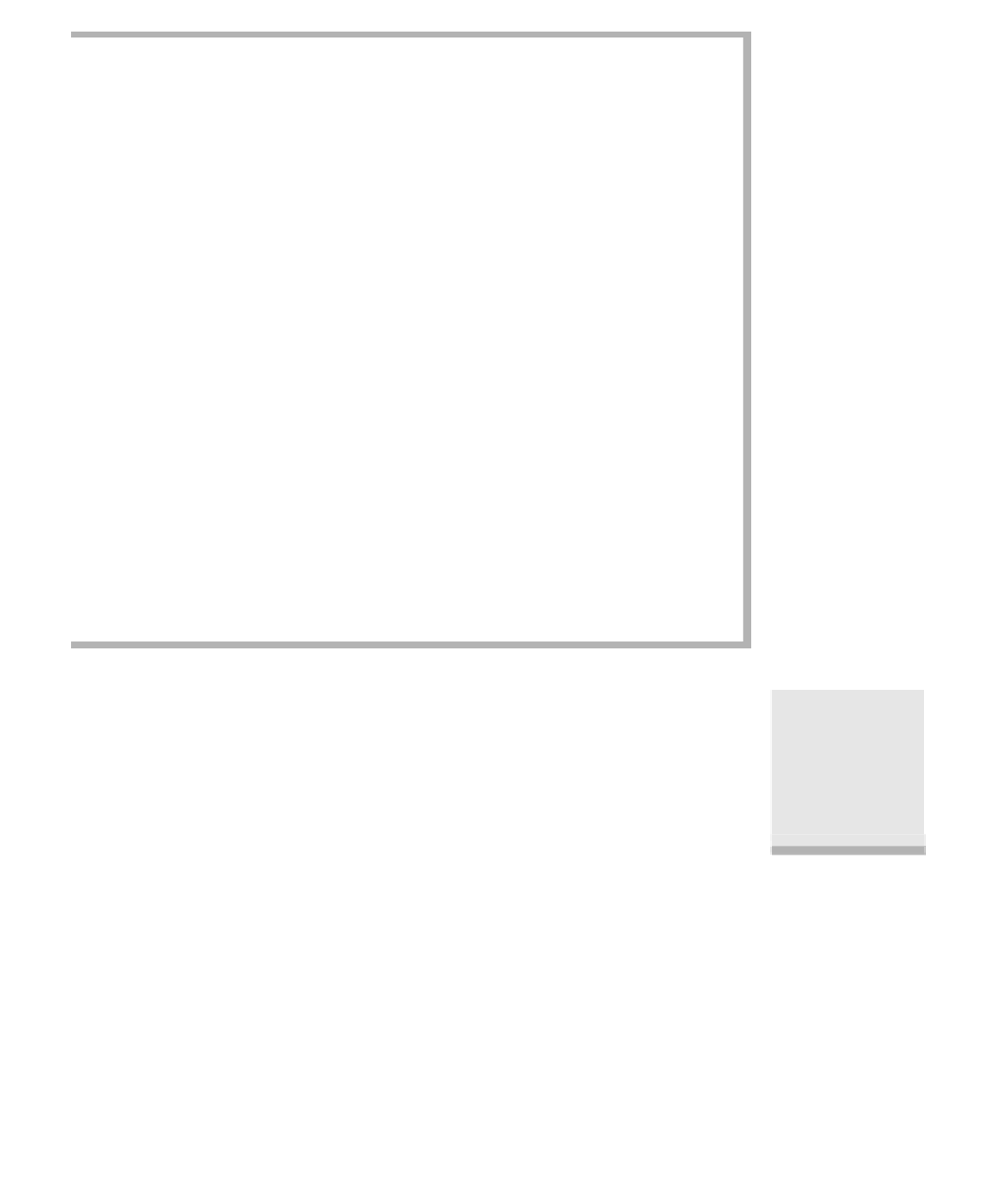
Search WWH ::

Custom Search