Game Development Reference
In-Depth Information
Note
Music plays a crucial role in the way players experience the environment and the action. Music can be
used to create tension, sadness, happiness, and many other emotions. However, dealing with music in games
is a lot harder than it is in movies. In movies, it's clear what is going to happen, so the music can match
perfectly. But in games, part of the action is under the player's control. Modern games use adaptive music
that constantly changes according to how the game story evolves.
If you want to implement more advanced handling of music and sound in your game, the basic JavaScript
high-level library for processing and synthesizing audio supported by many modern browsers.
In JavaScript, it's very easy to play background music or sound effects. To use sound, you first need
a sound file that you can play. In the FlyingSpriteWithSound program, you play the file
snd_music.mp3
,
which serves as background music. Similar to storing and using sprites, you add a variable to the
Game
object in which you store the music data. So, the
Game
object is declared and initialized as follows:
var Game = {
canvas : undefined,
canvasContext : undefined,
backgroundSprite : undefined,
balloonSprite : undefined,
balloonPosition : { x : 0, y : 50 },
backgroundMusic : undefined
};
You need to add a few instructions to the
start
method in order to load a sound effect or
background music. JavaScript provides a type that you can use as a blueprint for creating an object
that represents a sound. This type is called
Audio
. You can create an object of that type and begin
loading a sound as follows:
Game.backgroundMusic = new Audio();
Game.backgroundMusic.src = "snd_music.mp3";
As you can see, this works almost the same way as loading a sprite. Now you can call methods that
are defined as a part of this object, and you can set member variables of the object. For example,
the following instruction tells the browser to start playing the audio that is stored in the
Game.
backgroundMusic
variable:
Game.backgroundMusic.play();
You want to reduce the volume of the background music so you can play (louder) sound effects over
it later. Setting the volume is done with the following instruction:
Game.backgroundMusic.volume = 0.4;
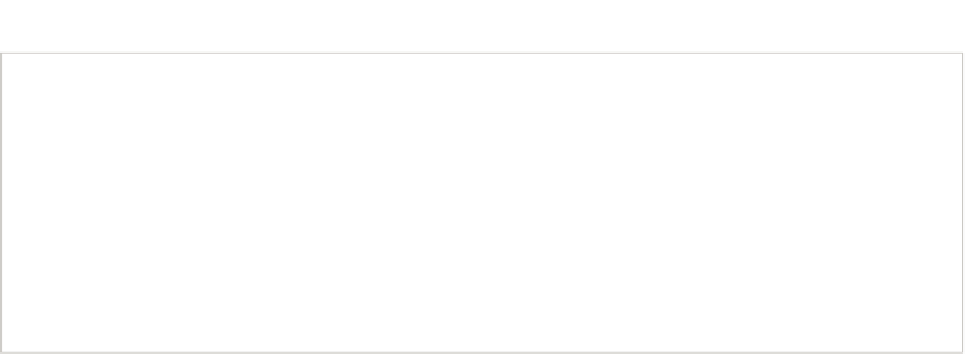
Search WWH ::

Custom Search