Information Technology Reference
In-Depth Information
Using Suggested Practices for Custom Attributes
When writing custom attributes, there are certain practices that are strongly suggested. They
include the following:
The attribute class should represent some state of the target construct.
If the attribute
requires
certain fields, include a constructor with positional parameters
to collect that data, and let optional fields be initialized with named parameters, as
needed.
Don't implement public methods or other function members other than properties.
For additional security, declare the attribute class as
sealed
.
Use the
AttributeUsage
attribute on your attribute declaration to explicitly specify the
set of attribute targets.
The following code illustrates these guidelines.
[AttributeUsage( AttributeTargets.Class )]
public sealed class MyAttributeAttribute : System.Attribute
{
private string _Description;
private string _VersionNumber;
private string _ReviewerID;
public string Description
{ get { return _Description; } set { _Description = value; } }
public string VersionNumber
{ get { return _VersionNumber; } set { _VersionNumber = value; } }
public string ReviewerID
{ get { return _ReviewerID; } set { _ReviewerID = value; } }
public MyAttributeAttribute(string desc, string ver)
{
Description = desc;
VersionNumber = ver;
}
}
Accessing an Attribute
Now that you know how to create custom attributes, you need to know how to access the meta-
data they contain. Suppose, for example, that you want to find out whether a particular
attribute is applied to a particular class, or you want a list of the attributes that are applied to a
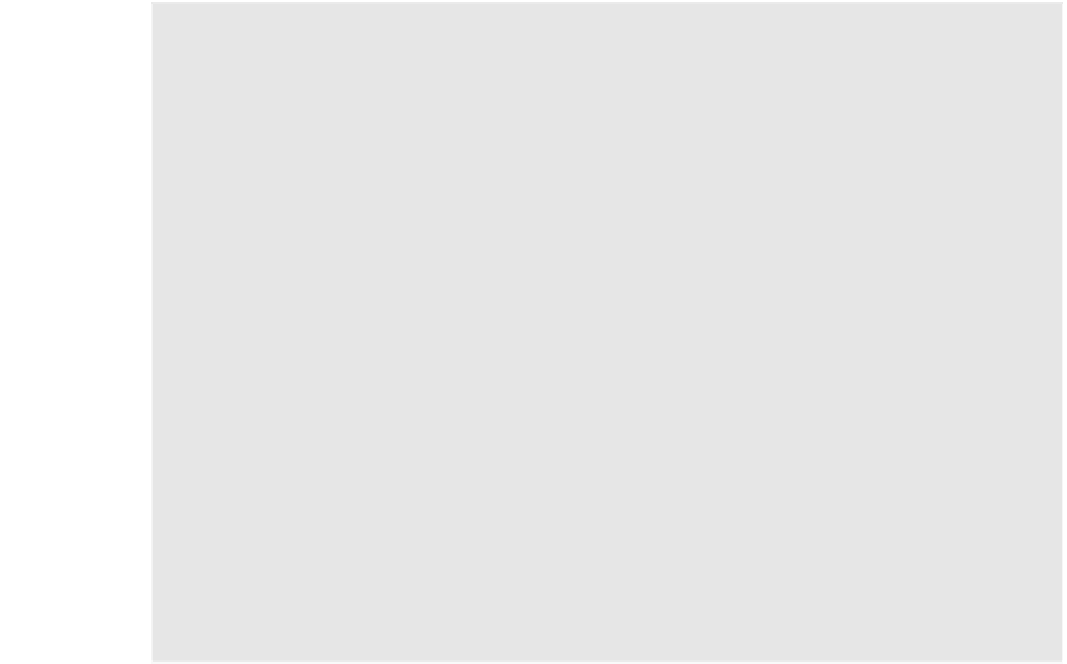


































































