Information Technology Reference
In-Depth Information
Now that
MyClass
implements
IComparable
,
Sort
will work on it as well. It would not, by the
way, have been sufficient to just declare the
CompareTo
method—it must be part of implement-
ing the interface, which means placing the interface name in the base class list.
The following shows the complete updated code, which can now use the
Sort
method
to sort an array of
MyClass
objects.
Main
creates and initializes an array of
MyClass
objects and
then prints them out. It then calls
Sort
and prints them out again to show that they have
been sorted.
class MyClass: IComparable // Class implements interface
{
public int TheValue;
public int CompareTo(object obj) // Implement the method.
{
MyClass mc = (MyClass)obj;
if (this.TheValue < mc.TheValue) return -1;
if (this.TheValue > mc.TheValue) return 1;
return 0;
}
}
class Program
{
static void PrintOut( string s, MyClass[] ma)
{
Console.Write(s);
foreach (MyClass i in ma)
Console.Write("{0} ", i.TheValue);
Console.WriteLine("");
}
static void Main()
{
int[] MyInt = new int[5] { 20, 4, 16, 9, 2 };
MyClass[] MyMc = new MyClass[5]; // Create array of MyClass objs
for (int i = 0; i < 5; i++) // Initialize the array.
{
MyMc[i] = new MyClass();
MyMc[i].TheValue = MyInt[i];
}
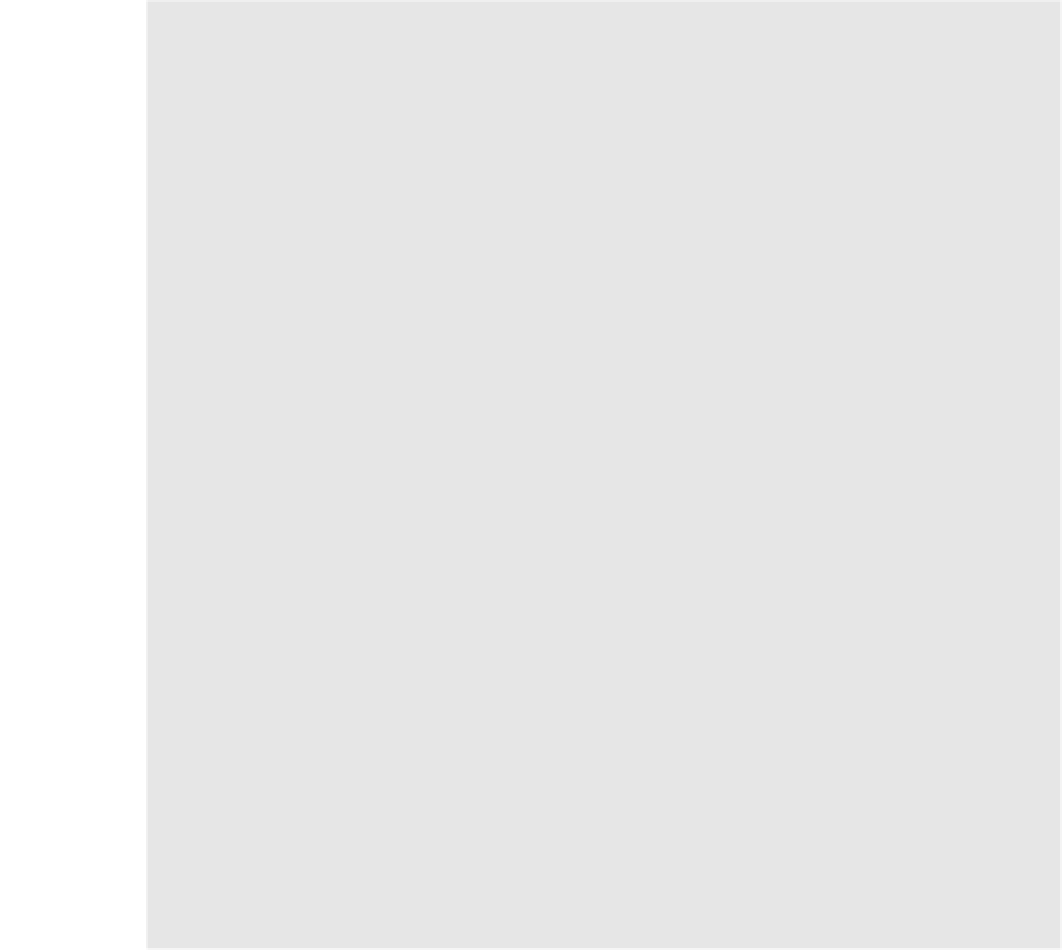


































































