Information Technology Reference
In-Depth Information
Delegate Example
The following code defines and uses a delegate with no parameters and no return value. Note
the following about the code:
Class
Test
defines two print functions.
Method
Main
creates an instance of the delegate and then adds three more methods.
The program then invokes the delegate, which calls its methods.
Note the test for the
null
delegate, before attempting to invoke it.
// Define a delegate with no return value and no parameters.
delegate void PrintFunction();
class Test
{
public void Print1()
{ Console.WriteLine("Print1 -- instance"); }
public static void Print2()
{ Console.WriteLine("Print2 -- static"); }
}
class Program
{
static void Main()
{
Test t = new Test(); // Create a test class instance.
PrintFunction pf; // Create a null delegate.
pf = t.Print1; // Instantiate and initialize the delegate.
// Add three more methods to the delegate.
pf += Test.Print2;
pf += t.Print1;
pf += Test.Print2;
// The delegate now contains four methods.
if( null != pf ) // Make sure the delegate has methods.
pf(); // Invoke the delegate.
else
Console.WriteLine("Delegate is empty");
}
}
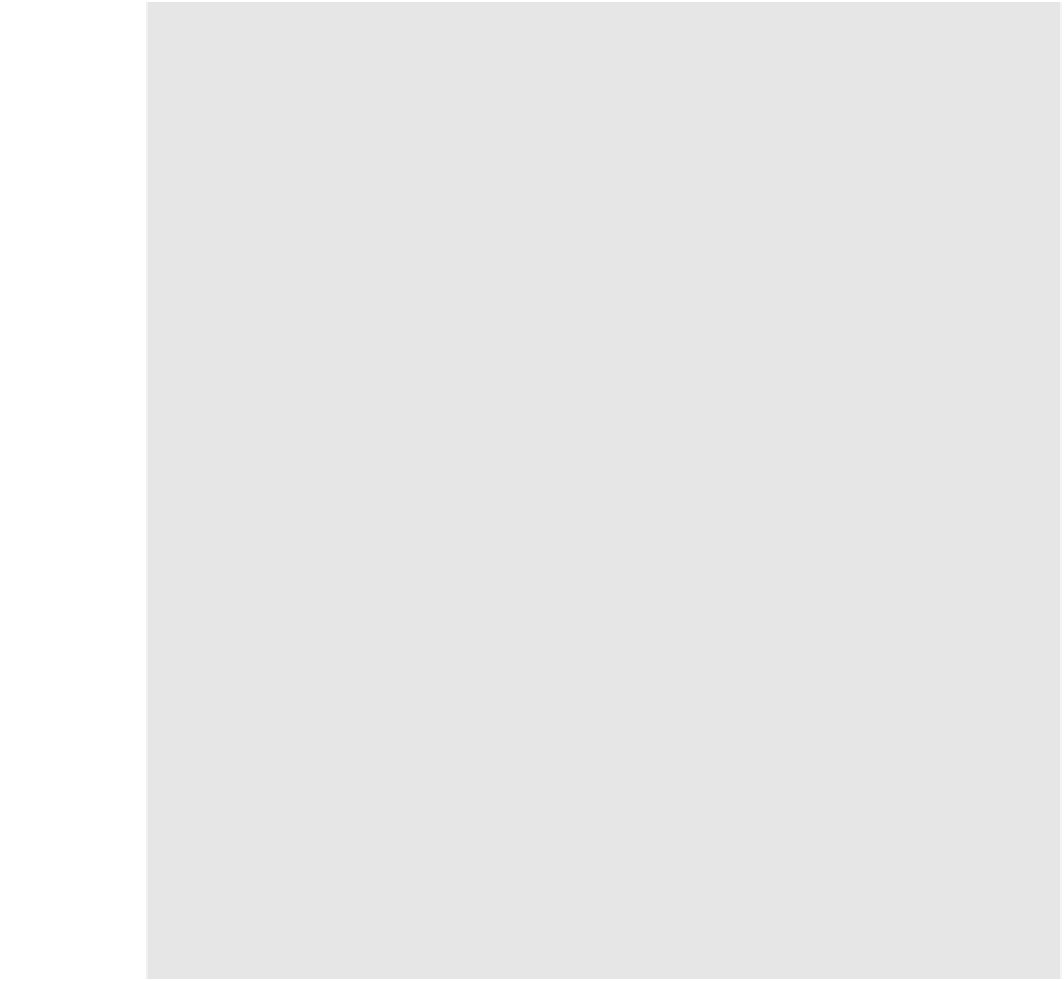


































































