Information Technology Reference
In-Depth Information
The following are some examples:
bool bVal;
bVal = (1 == 1) && (2 == 2); // True, both operand expressions are true
bVal = (1 == 1) && (1 == 2); // False, second operand expression is false
bVal = (1 == 1) || (2 == 2); // True, both operand expressions are true
bVal = (1 == 1) || (1 == 2); // True, first operand expression is true
bVal = (1 == 2) || (2 == 3); // False, both operand expressions are false
bVal = true; // Set bVal to true.
bVal = !bVal; // bVal is now false.
The conditional logical operators operate in “short circuit” mode, meaning that, if after
evaluating
Expr1
the result can already be determined, then it skips the evaluation of
Expr2
.
The following code shows examples of expressions in which the value can be determined after
evaluating the first operand:
bool bVal;
bVal = (1 == 2) && (2 == 2); // False, after evaluating first expression
bVal = (1 == 1) || (1 == 2); // True, after evaluating first expression
Because of the short circuit behavior, do not place expressions with side effects (such as
changing a value) in
Expr2
, since they might not be evaluated. In the following code, the post-
increment of variable
iVal
would not be executed.
bool bVal; int iVal = 10;
bVal = (1 == 2) && (9 == iVal++); // result: bVal = False, iVal = 10
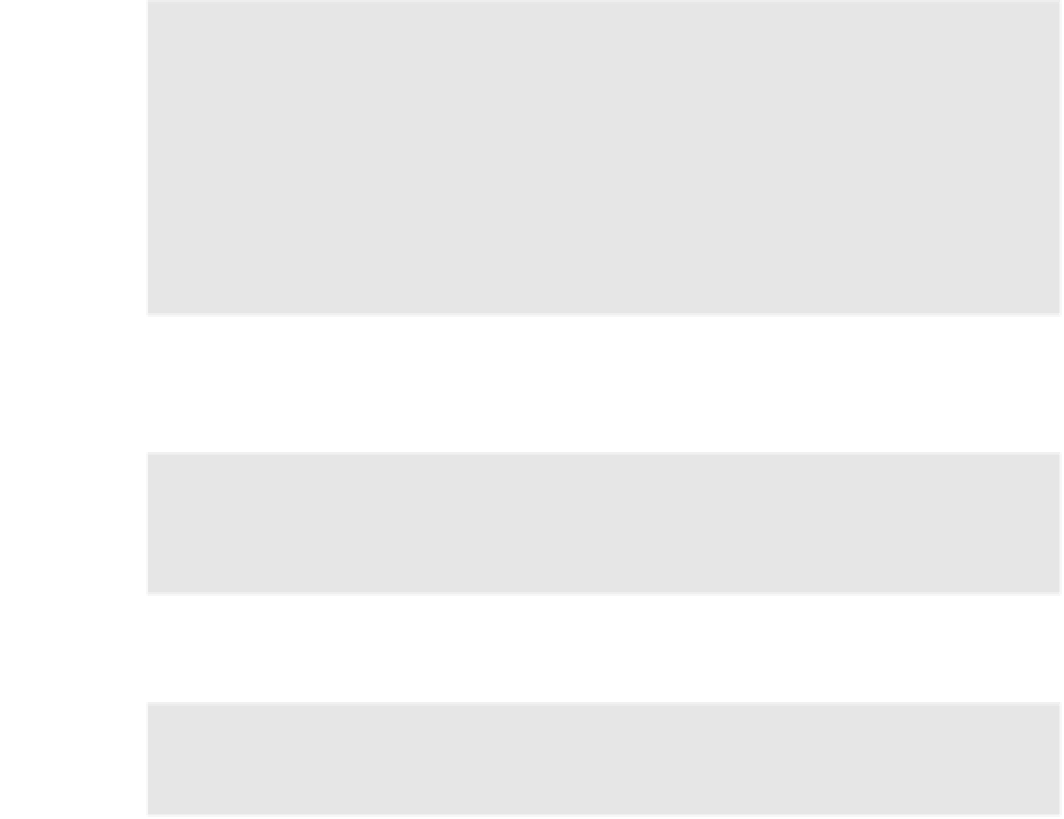


































































