Information Technology Reference
In-Depth Information
For example, in the following code, the values of
MyField1
and
MyField2
would be set to 5
and 0 respectively, before the base class constructor was called.
class MyDerivedClass : MyBaseClass
{
int MyField1 = 5; // 1. Member initialized.
int MyField2; // Member initialized.
public MyDerivedClass() // 3. Body of constructor executed.
{
...
}
}
class MyBaseClass
{
public MyBaseClass() // 2. Base class constructor called.
{
...
}
}
■
Caution
Calling a virtual method in a constructor is
strongly discouraged
. The virtual method in the base
class would call the override method in the derived class while the base class constructor was being exe-
cuted. But that would be before the derived constructor's body was executed. It would, therefore, be calling
up into the derived class before the class was completely initialized.
Constructor Initializers
By default, the parameterless constructor of the base class is called when an object is being
constructed. But constructors can be overloaded, so a base class might have more than one. If
you want your derived class to use a specific base class constructor other than the parameter-
less constructor, you must specify it in a
constructor initializer
.
There are two forms of constructor initializer:
The first form uses the keyword
base
and specifies which base class constructor to use.
The second form uses the keyword
this
and specifies which other constructor from
this
class should be used.
A base class constructor initializer is placed after a colon following the parameter list in a
class's constructor declaration. The constructor initializer consists of the keyword
base
, and
the parameter list of the base constructor to call.
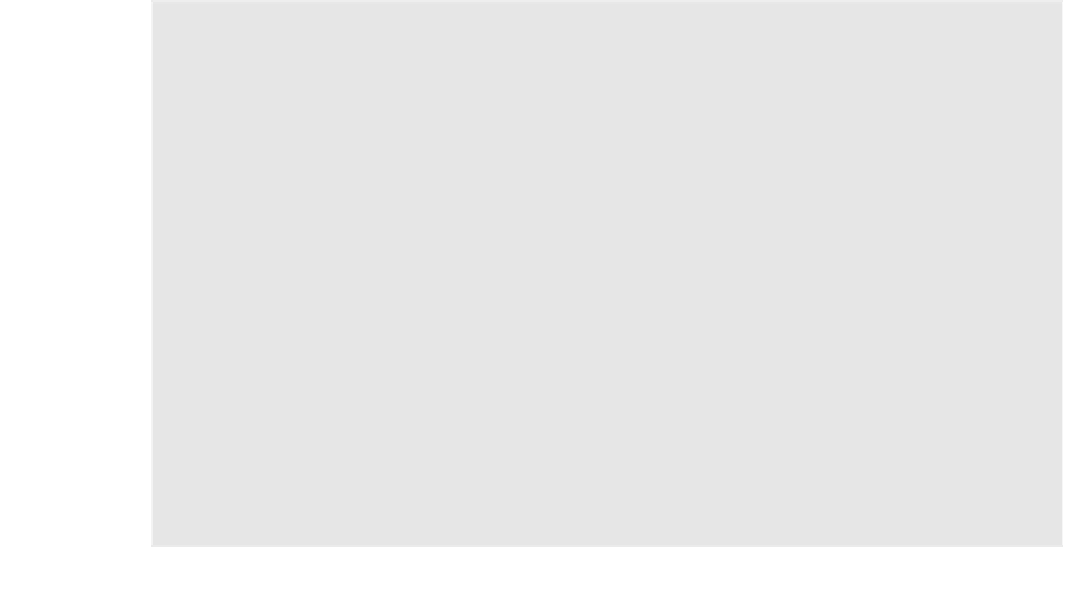




































































