Information Technology Reference
In-Depth Information
Parameters
So far, you have seen that methods are named units of code that can be called from many
places in a program, and can return a single value to the calling code. Returning a single value
is certainly valuable, but what if you need to return multiple values? Also, it would be useful to
be able to pass data into a method when it starts execution.
Parameters
are special variables
that allow you to do both these things.
Formal Parameters
Formal parameters
are local variables that are declared in the method's parameter list, rather
than in the body of the method.
The following method header shows the syntax of parameter declarations. It declares two
formal parameters—one of type
int
, and the other of type
float
.
public void PrintSum(
int x, float y
)
{ ... }
↑
Formal parameter declarations
Because formal parameters are variables, they have a data type and a name, and can be
written to and read from.
Unlike a method's other local variables, the parameters are defined outside the method
body and initialized before the method starts, except in one case, which I will cover
shortly.
The parameter list can have any number of formal parameter declarations, and the dec-
larations must be separated by commas.
The formal parameters are used throughout the method body, for the most part, just like
other local variables. For example, the following declaration of method
PrintSum
uses two for-
mal parameters,
x
and
y
, and a local variable,
Sum
, all of type
int
.
public void PrintSum( int x, int y )
{
int Sum = x + y;
Console.WriteLine("Newsflash: {0} + {1} is {2}", x, y, Sum);
}
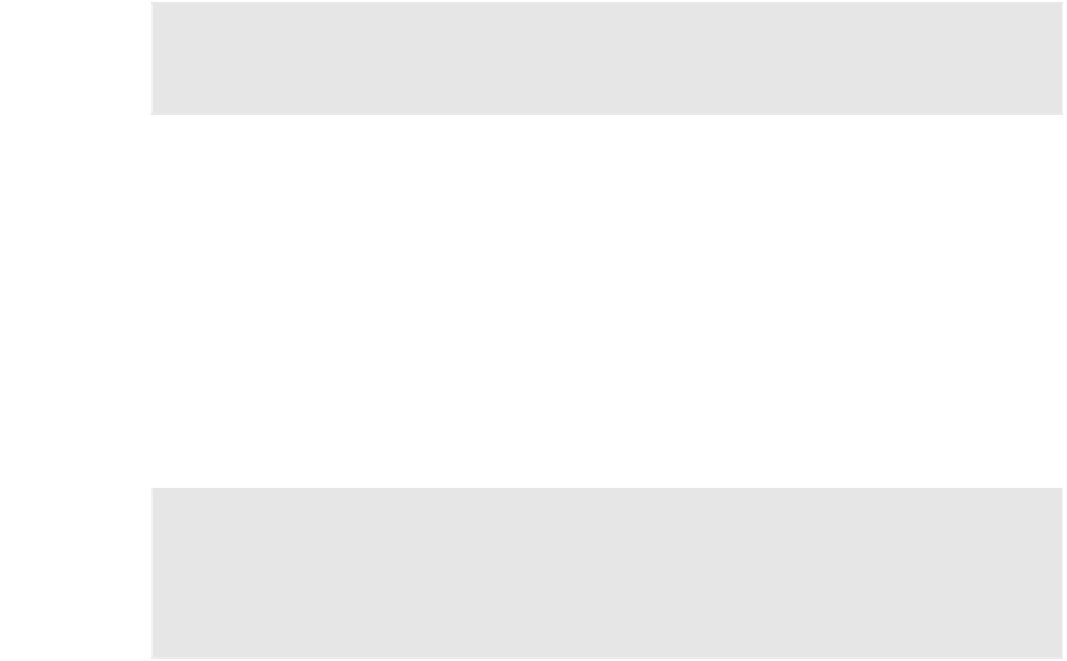


































































