Game Development Reference
In-Depth Information
The C++ Stack Memory Model
The stack is more difficult to understand. Every time you call a function, the compiler generates code
behind the scenes to allocate memory for the parameters and local variables for the function being
called. Listing 22-1 shows some simple code that we then use to explain how the stack operates.
Listing 22-1. A Simple C++ Program
void function2(int variable1)
{
int variable2{ variable1 };
}
void function1(int variable)
{
function2(variable);
}
int _tmain(int argc, _TCHAR* argv[])
{
int variable{ 0 };
function1(variable);
return 0;
}
The program in Listing 22-1 is very simple: It begins with
_tmain
, which calls
function1
which calls
function2
. Figure
22-1
illustrates what the stack would look like for the main function.
_tmain: variable= 0
Figure 22-1.
The stack for
_tmain
The stack space for
main
is very simple. It has a single storage space for the local variable named
variable
. These stack spaces for individual functions are known as
stack frames
. When
function1
is
called, a new stack frame is created on top of the existing frame for
_tmain
. Figure
22-2
shows this
in action.
function1.variable = _tmain.variable
_tmain.variable = 0
Figure 22-2.
The added stack frame for
function1


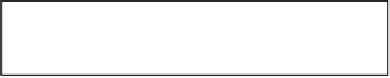