Java Reference
In-Depth Information
System
.
out
.
println
(
question
+
" ("
+
responses
[
0
]
+
"/"
+
responses
[
1
]
+
" ): "
);
// Both the array reference and the array index may be more complex
double
datum
=
data
.
getMatrix
()[
data
.
row
()
*
data
.
numColumns
()
+
data
.
column
()];
The array index expression must be of type
int
, or a type that can be widened to an
int
:
byte
,
short
, or even
char
. It is obviously not legal to index an array with a
boolean
,
float
, or
double
value. Remember that the
length
field of an array is an
int
and that arrays may not have more than
Integer.MAX_VALUE
elements. Index‐
ing an array with an expression of type
long
generates a compile-time error, even if
the value of that expression at runtime would be within the range of an
int
.
a
x
Array bounds
Remember that the first element of an array
a
is
a[0]
, the second element is
a[1]
,
and the last is
a[a.length-1]
.
A common bug involving arrays is use of an index that is too small (a negative
index) or too large (greater than or equal to the array
length
). In languages like C
or C++, accessing elements before the beginning or after the end of an array yields
unpredictable behavior that can vary from invocation to invocation and platform to
platform. Such bugs may not always be caught, and if a failure occurs, it may be at
some later time. While it is just as easy to write faulty array indexing code in Java,
Java guarantees predictable results by checking every array access at runtime. If an
array index is too small or too large, Java immediately throws an
ArrayIndexOutOf
BoundsException
.
Iterating arrays
It is common to write loops that iterate through each of the elements of an array in
order to perform some operation on it. This is typically done with a
for
loop. The
following code, for example, computes the sum of an array of integers:
int
[]
primes
=
{
2
,
3
,
5
,
7
,
11
,
13
,
17
,
19
,
23
};
int
sumOfPrimes
=
0
;
for
(
int
i
=
0
;
i
<
primes
.
length
;
i
++)
sumOfPrimes
+=
primes
[
i
];
The structure of this
for
loop is idiomatic, and you'll see it frequently. Java also has
the foreach syntax that we've already met. The summing code could be rewritten
succinctly as follows:
for
(
int
p
:
primes
)
sumOfPrimes
+=
p
;
Copying arrays
All array types implement the
Cloneable
interface, and any array can be copied by
invoking its
clone()
method. Note that a cast is required to convert the return value

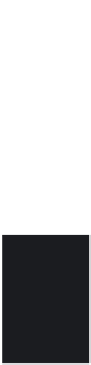