Java Reference
In-Depth Information
In this example, the inner
if
statement forms the single statement allowed by the
syntax of the outer
if
statement. Unfortunately, it is not clear (except from the hint
given by the indentation) which
if
the
else
goes with. And in this example, the
indentation hint is wrong. The rule is that an
else
clause like this is associated with
the nearest
if
statement. Properly indented, this code looks like this:
a
x
if
(
i
==
j
)
if
(
j
==
k
)
System
.
out
.
println
(
"i equals k"
);
else
System
.
out
.
println
(
"i doesn't equal j"
);
// WRONG!!
This is legal code, but it is clearly not what the programmer had in mind. When
working with nested
if
statements, you should use curly braces to make your code
easier to read. Here is a better way to write the code:
if
(
i
==
j
)
{
if
(
j
==
k
)
System
.
out
.
println
(
"i equals k"
);
}
else
{
System
.
out
.
println
(
"i doesn't equal j"
);
}
The else if clause
The
if/else
statement is useful for testing a condition and choosing between two
statements or blocks of code to execute. But what about when you need to choose
between several blocks of code? This is typically done with an
else
if
clause, which
is not really new syntax, but a common idiomatic usage of the standard
if/else
statement. It looks like this:
if
(
n
==
1
)
{
// Execute code block #1
}
else
if
(
n
==
2
)
{
// Execute code block #2
}
else
if
(
n
==
3
)
{
// Execute code block #3
}
else
{
// If all else fails, execute block #4
}
There is nothing special about this code. It is just a series of
if
statements, where
each
if
is part of the
else
clause of the previous statement. Using the
else
if
idiom
is preferable to, and more legible than, writing these statements out in their fully
nested form:
if
(
n
==
1
)
{
// Execute code block #1

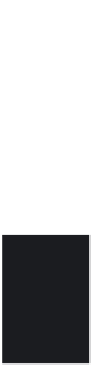