Java Reference
In-Depth Information
The desire to add new methods to existing interfaces was
directly responsible for the new language feature referred to as
default methods
(see
“Default Methods” on page 140
for more
details). Without this new mechanism, older implementations
of the Collections interfaces would fail to compile under Java
8, and would fail to link if loaded into a Java 8 runtime.
In this section, we will give a basic introduction to the use of lambda expressions in
the Java Collections. For a fuller treatment, see
Java 8 Lambdas
by Richard Warbur‐
ton (O'Reilly).
Functional Approaches
The approach that Java 8 wished to enable was derived from functional program‐
ming languages and styles. We met some of these key patterns in
“Method Refer‐
ences” on page 173
—let's reintroduce them and look at some examples of each.
Filter
The idiom applies a piece of code (that returns either true or false) to each element
in a collection, and builds up a new collection consisting of those elements that
“passed the test" (i.e., the bit of code returned true when applied to the element).
For example, let's look at some code to work with a collection of cats and pick out
the tigers:
String
[]
input
=
{
"tiger"
,
"cat"
,
"TIGER"
,
"Tiger"
,
"leopard"
};
List
<
String
>
cats
=
Arrays
.
asList
(
input
);
String
search
=
"tiger"
;
String
tigers
=
cats
.
stream
()
.
filter
(
s
->
s
.
equalsIgnoreCase
(
search
))
.
collect
(
Collectors
.
joining
(
", "
));
System
.
out
.
println
(
tigers
);
The key piece is the call to
filter()
, which takes a lambda expression. The lambda
takes in a string, and returns a Boolean value. This is applied over the whole collec‐
tion
cats
, and a new collection is created, which only contains tigers (however they
were capitalized).
The
filter()
method takes in an instance of the
Predicate
interface, from the new
package
java.util.function
. This is a functional interface, with only a single non‐
default method, and so is a perfect fit for a lambda expression.
s
a
Note the final call to
collect()
; this is an essential part of the API and is used to
“gather up” the results at the end of the lambda operations. We'll discuss it in more
detail in the next section.
Predicate
has some other very useful default methods, such as for constructing
combined predicates by using logic operations. For example, if the tigers want to
admit leopards into their group, this can be represented by using the
or()
method:
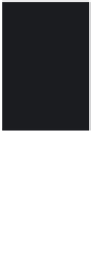
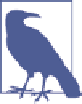