Java Reference
In-Depth Information
Extending a Class
In
Example 3-3
, we show how we can implement
PlaneCircle
as a subclass of the
Circle
class.
Example 3-3. Extending the Circle class
public
class
PlaneCircle
extends
Circle
{
// We automatically inherit the fields and methods of Circle,
// so we only have to put the new stuff here.
// New instance fields that store the center point of the circle
private
final
double
cx
,
cy
;
m
g
// A new constructor to initialize the new fields
// It uses a special syntax to invoke the Circle() constructor
public
PlaneCircle
(
double
r
,
double
x
,
double
y
)
{
super
(
r
);
// Invoke the constructor of the superclass, Circle()
this
.
cx
=
x
;
// Initialize the instance field cx
this
.
cy
=
y
;
// Initialize the instance field cy
}
O
public
double
getCentreX
()
{
return
cx
;
}
public
double
getCentreY
()
{
return
cy
;
}
// The area() and circumference() methods are inherited from Circle
// A new instance method that checks whether a point is inside the circle
// Note that it uses the inherited instance field r
public
boolean
isInside
(
double
x
,
double
y
)
{
double
dx
=
x
-
cx
,
dy
=
y
-
cy
;
// Distance from center
double
distance
=
Math
.
sqrt
(
dx
*
dx
+
dy
*
dy
);
// Pythagorean theorem
return
(
distance
<
r
);
// Returns true or false
}
}
Note the use of the keyword
extends
in the first line of
Example 3-3
. This keyword
tells Java that
PlaneCircle
extends, or subclasses,
Circle
, meaning that it inherits
the fields and methods of that class.
There are several different ways to express the idea that our
new object type has the characteristics of a
Circle
as well as
having a position. This is probably the simplest, but is not
always the most suitable, especially in larger systems.


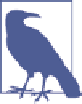