Java Reference
In-Depth Information
location 1000
table = 1000
1
1 2
1 2 3
FIGURE 5.6:
Example of ragged array.
being able to easily manipulate it. For example, consider a method that takes as input a
two-dimensional array and computes the sum of the elements in it. Here is one possible
implementation.
public static int
sum(
int
[][] a)
{
int
sum = 0 ;
for
(
int
row = 0; row
<
a . l e n g t h ; row++)
{
for
(
int
col = 0; col
<
a [ row ] . l e n g t h ;
c o l ++)
{
sum += a [ row ] [ c o l ] ;
}
return
sum ;
}
The first
for
loop iterates through all the rows. Note that
a.length
will return the
number of rows. Alternatively,
a[0].length
returns the numbers of elements in the first
row of the two-dimensional array. Therefore, the above code will compute the sum of the
elements in a two-dimensional array even when the array is ragged. Remember that every
time we are only reading an array without modifying it, we can use a for-each
for
loop to
access it. Accordingly, the above code can be rewritten as shown below.
public static int
sum(
int
[][] a)
{
int
sum = 0 ;
for
(
int
[] row: a)
{
for
(
int
element : row)
{
sum += element ;
}
return
sum ;
}
Now the first loop iterates over all rows. This is possible because the rows in a two-
dimensional array are stored as arrays themselves. The second loop simply iterates though
all the elements of the current row. Note that both implementations perform correctly on
ragged arrays.
Recall that a one-dimensional array can be declared and populated in a single statement
using the following syntax.
int
[] a =
{
5,8,7
}
;
The same applies for a two-dimensional array. For example, the ragged array from Fig-
ure 5.6 can be created using the following statement.
int
[][] table =
{{
1
}
,
{
1,2
}
,
{
1,2,3
}}
;
Here,
table.length
will return 3 because there are three rows. Conversely,




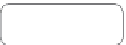







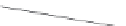









