Java Reference
In-Depth Information
if(hypotenuse == 0.0) // Make sure it's
hypotenuse = 1.0; // non-zero
// Angle is the arc sine of perp/hypotenuse. Clockwise is positive angle
return -Line2D.relativeCCW(position.x, position.y,
start.x, start.y,
last.x, last.y)*Math.asin(perp/hypotenuse);
}
This is basically just an assembly of the code fragments calculating the angle in the last section. We will
need an import statement for
Line2D
for
SketchView
:
import java.awt.geom.Line2D;
We completed the
mouseReleased()
method when we dealt with
MOVE
mode so there's nothing
further to add there.
Now we must empower our element classes to rotate themselves. We will add a data member to the
base class to store the rotation angle, and a method to rotate the element:
public abstract class Element {
public Element(Color color) {
this.color = color; }
public Color getColor() {
return color; }
// Set or reset highlight color
public void setHighlighted(boolean highlighted) {
this.highlighted = highlighted;
}
public Point getPosition() {
return position;
}
protected void draw(Graphics2D g2D, Shape element) {
g2D.setPaint(highlighted ? Color.MAGENTA : color); // Set the element color
AffineTransform old = g2D.getTransform(); // Save the current transform
g2D.translate(position.x, position.y); // Translate to position
g2D.rotate(angle); // Rotate about position
g2D.draw(element); // Draw the element
g2D.setTransform(old); // Restore original transform
}
protected java.awt.Rectangle getBounds(java.awt.Rectangle bounds) {
AffineTransform at = AffineTransform.getTranslateInstance(
position.x, position.y);
at.rotate(angle);
return at.createTransformedShape(bounds).getBounds();
}
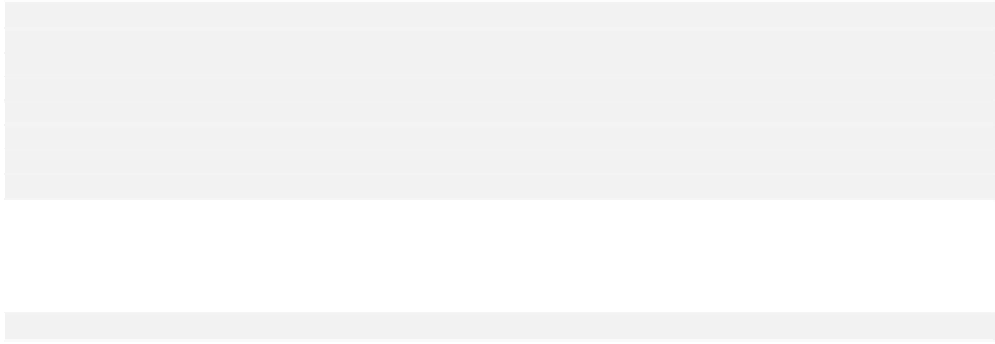






