Java Reference
In-Depth Information
It's not going to be too difficult. We can arbitrarily decide that an element is under the cursor when the
cursor position is inside the bounding rectangle for an element. This is not too precise a method, but it
has the great attraction that it is extremely simple. Precise hit-testing on an element would carry
considerably more processing overhead. Electing to add any greater complexity will not help us to
understand the principles here, so we will stick with the simple approach.
So what is going to be the methodology for finding the element under the cursor? Brute force basically:
whenever the mouse is moved, we can just search through the bounding rectangles for each of the
elements in the document until we find one that encloses the current cursor position. We will then
arrange for the first element that we find to be highlighted. If we get right through all the elements in
the document without finding a bounding rectangle that encloses the cursor, then there isn't an element
under the cursor.
To record a reference to the element that is under the cursor, we will add a data member of type
Element
to the
SketchView
class. If there isn't an element under the cursor, we will make sure that
this data member is
null
.
Try It Out - Referring to Elements
Add the following statement after the statement declaring the
theApp
data member in the
SketchView
class definition:
private Element highlightElement; // Highlighted element
The
mouseMoved()
method is going to be called very frequently, so we need to make sure it executes
as quickly as possible. This means that for any given set of conditions, we execute the minimum amount
of code. Here's the implementation of the
mouseMoved()
method in the
MouseHandler
class in
SketchView
:
// Handle mouse moves
public void mouseMoved(MouseEvent e) {
Point currentCursor = e.getPoint(); // Get current cursor position
Iterator elements = theApp.getModel().getIterator();
Element element = null; // Stores an element
while(elements.hasNext()) { // Go through the list
element = (Element)elements.next(); // Get the next element
if(element.getBounds().contains(currentCursor)) { // Under the cursor?
if(element==highlightElement) // If it's already highlighted
return; // we are done
g2D = (Graphics2D)getGraphics(); // Get graphics context
if(highlightElement!=null) { // If an element is highlighted
highlightElement.setHighlighted(false);// un-highlight it and
highlightElement.draw(g2D); // draw it normal color
}
element.setHighlighted(true); // Set highlight for new element
highlightElement = element; // Store new highlighted element
element.draw(g2D); // Draw it highlighted
g2D.dispose(); // Release graphic context resources
g2D = null;
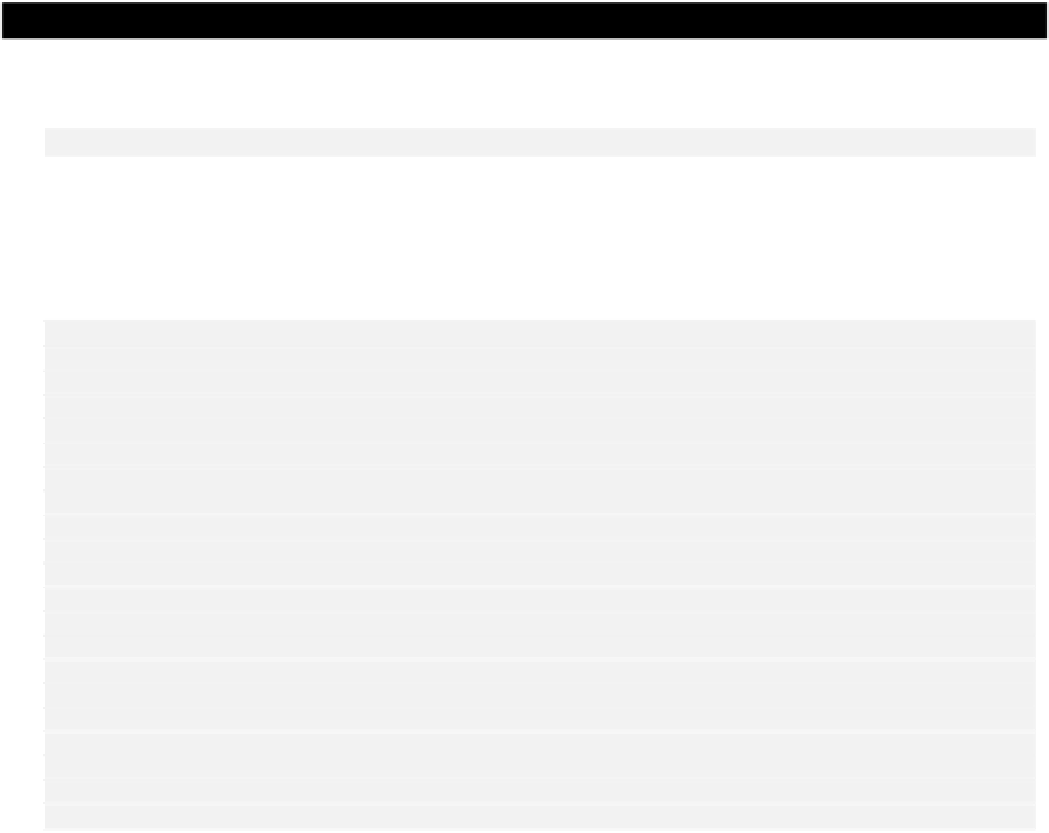









