Java Reference
In-Depth Information
It looks like a lot of code but it's repetitive as we have two radio buttons. The second argument to the
JRadioButton
constructor sets the state of the button. If the existing style of the current font is BOLD
and/or
ITALIC
, the initial states of the buttons will be set accordingly. We add a listener of type
StyleListener
for each button and we will add this as an inner class to
FontDialog
in a moment. Note
that we pass the style constant that corresponds to the set state of the button to the constructor for the listener.
The
stylePane
object presents the buttons using the default
FlowLayout
manager, and this pane is added
as the last row to
dataPane
. The final step is to add the
dataPane
object as the central pane in the content
pane for the dialog. The call to
pack()
lays out the dialog components with their preferred sizes if possible,
and the
setVisible()
call with the argument
false
means that the dialog is initially hidden. Since this is
a complex dialog we won't want to create a new object each time we want to display the font dialog. We will
just call the
setVisible()
method for the dialog object with the argument
true
.
Listening for Radio Buttons
The inner class,
StyleListener
, in the
FontDialog
class will work on principles that you have seen
before. A radio button (or a checkbox) generates
ItemEvent
events and the listener class must
implement the
ItemListener
interface:
class StyleListener implements ItemListener {
public StyleListener(int style) {
this.style = style;
}
public void itemStateChanged(ItemEvent e) {
if(e.getStateChange()==ItemEvent.SELECTED) // If style was selected
fontStyle |= style; // turn it on in the font style
else
fontStyle &= ~style; // otherwise turn it off
font = font.deriveFont(fontStyle); // Get a new font
fontDisplay.setFont(font); // Change the label font
fontDisplay.repaint(); // repaint
}
private int style; // Style for this listener
}
The constructor accepts an argument that is the style for the button, so the value of the member,
style
,
will be the value we want to set in the
fontStyle
member that we use to create a new
Font
object,-
either
Font.BOLD
or
Font.ITALIC
. Since the listener for a particular button already contains the
corresponding style, the
itemStateChanged()
method that is called when an item event occurs just
switches the value of
style
in the
fontStyle
member of
FontDialog
either on or off, dependent
on whether the radio button was selected or deselected. It then derives a font with the new style, sets it
in the
fontDisplay
label and repaints it.
We have now completed the
FontDialog
class. If you have been creating the code yourself, now
would be a good time to try compiling the class. All we need now is some code in the
SketchFrame
class to make use of it.
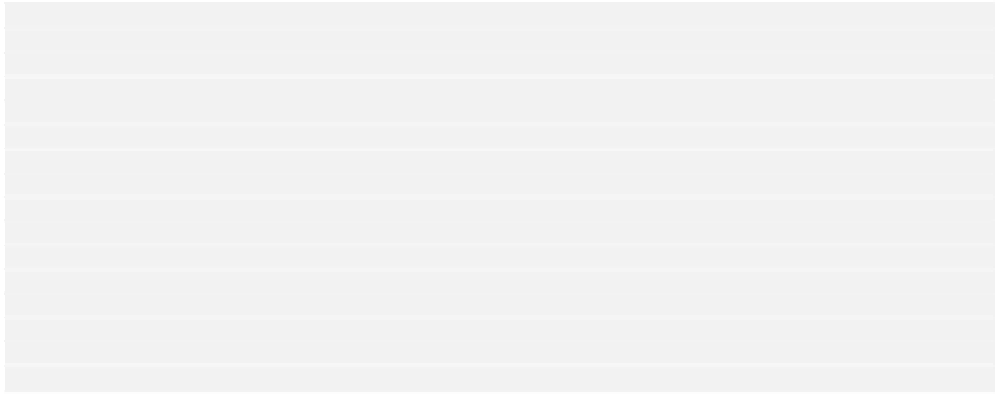




