Java Reference
In-Depth Information
// Nested class defining a line
public static class Line extends Element {
public Line(Point start, Point end, Color color) {
super(color);
line = new Line2D.Double(start, end);
}
public Shape getShape() {
return line;
}
public java.awt.Rectangle getBounds() {
return line.getBounds();
}
public void modify(Point start, Point last) {
line.x2 = last.x;
line.y2 = last.y;
}
private Line2D.Double line;
}
}
We have to specify the
Line
class as
static
to avoid a dependency on an
Element
object being
available. The
Element
class is abstract so there's no possibility of creating objects of this type. The
constructor has three parameters, the two end points of the line as type
Point
and the color.
Point
arguments to the constructor can be of type
Point2D
or type
Point
as
Point2D
is a superclass of
Point
. After passing the color to the base class constructor, we create the line as a
Line2D.Double
object. Since this implements the
Shape
interface, we can return it as type
Shape
from the
getShape()
method.
The
getBounds()
method couldn't be simpler. We just return the
Rectangle
object produced by the
getBounds()
method for the object,
line
. However, note how we have fully qualified the return type.
This is because we will be adding a
Rectangle
class as a nested class to the
Element
class. When we do,
the compiler will interpret the type
Rectangle
here as our rectangle class, and not the one defined in the
java.awt
package. You can always use a fully qualified class name when conflicts like this arise.
Try It Out - Drawing Lines
If you have saved the
Element
class definition as
Element.java
in the same directory as the rest of
the Sketcher classes, all you need to do is make sure all the constructor calls other than
Element.Line
are commented out in the
createElement()
member of the
MouseHandler
class, that is an inner
class to
SketchView
. The code for the method should look like this:
private Element createElement(Point start, Point end) {
switch(theApp.getWindow().getElementType()) {
case LINE:
return new Element.Line(start, end,
theApp.getWindow().getElementColor());
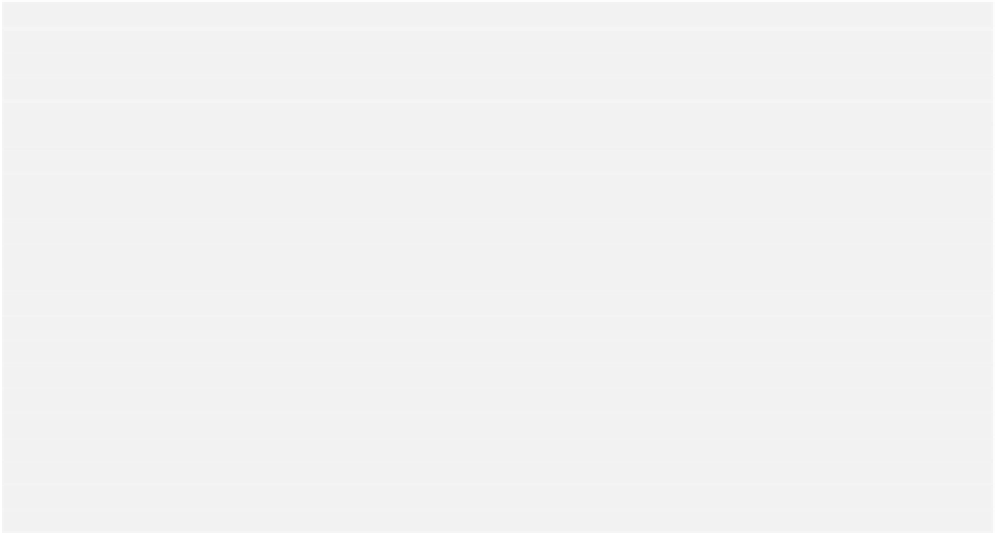
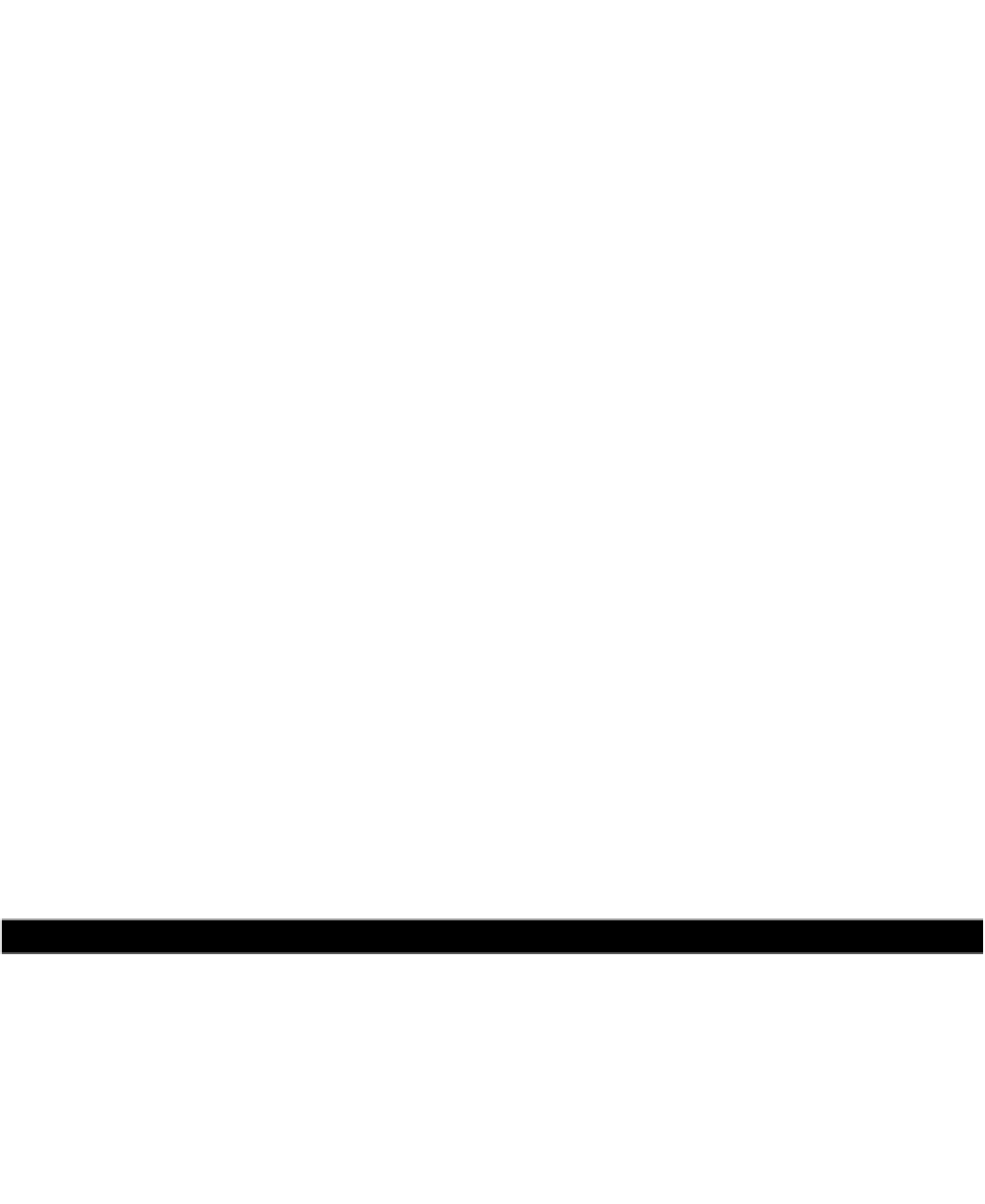







