Java Reference
In-Depth Information
float delta = 60; // Increment between stars
float starty = 0; // Starting y position
// Draw 3 rows of 4 stars
for(int yCount = 0 ; yCount<3; yCount++) {
starty += delta; // Increment row position
float startx = 0; // Start x position in a row
// Draw a row of 4 stars
for(int xCount = 0 ; xCount<4; xCount++) {
g2D.setPaint(Color.BLUE); // Drawing color blue
g2D.draw(star.atLocation(startx += delta, starty));
g2D.setPaint(Color.GREEN); // Color for fill is green
g2D.fill(star.getShape()); // Fill the star
}
}
}
We also need an import statement for the
Color
class name:
import java.awt.Color;
Now the applet window will look something like that
shown here - but in color of course.
How It Works
We set the color for drawing and filling the stars separately, simply to show that we can get both. The
stars are displayed in green with a blue boundary. You can fill a shape without drawing it - just call the
fill()
method. You could amend the example to do this by modifying the inner loop to:
for(int xCount = 0 ; xCount<4; xCount++) {
g2D.setPaint(Color.GREEN); // Color for fill is green
g2D.fill(star.atLocation(startx += delta, starty)); // Fill the star
}
Now all we will get is the green fill for each shape - no outline.


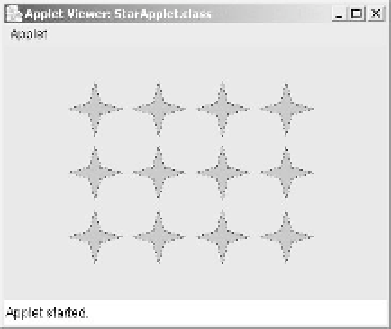













