Java Reference
In-Depth Information
Combining Rectangles
You can combine two rectangles to produce a new rectangle that is either the
union
of the two original
rectangles or the
intersection
. Let's take a couple of specifics to see how this works. We can create two
rectangles with the statements:
float width = 120.0f;
float height = 90.0f;
Rectangle2D.Float rect1 = new Rectangle2D.Float(50.0f, 150.0f, width, height);
Rectangle2D.Float rect2 = new Rectangle2D.Float(80.0f, 180.0f, width, height);
We can obtain the intersection of the two rectangles with the statement:
Rectangle2D.Float rect3 = rect1.createIntersection(rect2);
The effect is illustrated in the diagram below by the shaded rectangle. Of course, the result is the same if
we call the method for
rect2
with
rect1
as the argument. If the rectangles don't overlap the rectangle
that is returned will be the rectangle from the bottom right of one rectangle to the top right of the other
that does not overlap either.
The following statement produces the union of the two rectangles:
Rectangle2D.Float rect3 = rect1.createUnion(rect2);
The result is shown in the diagram
by the rectangle with the heavy
boundary that encloses the other
two.
Testing Rectangles
Perhaps the simplest test you can apply is for an empty rectangle. The
isEmpty()
method that is
implemented in all the rectangle classes returns
true
if the
Rectangle2D
object is empty - which is
when either the width or the height (or both) are zero.
You can also test whether a point lies inside any type of rectangle object by calling its
contains()
method. There are
contains()
methods for all the rectangle classes that accept a
Point2D
argument or a pair of (
x
,
y
) coordinates of a type matching that of the rectangle class: they
return
true
if the point lies within the rectangle. Each shape class defines a
getBounds2D()
method
that returns a
Rectangle2D
object that encloses the shape.
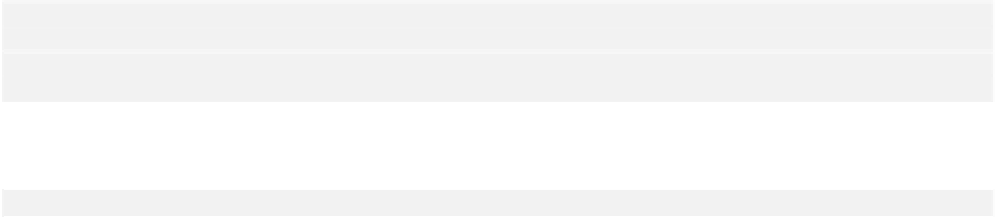




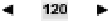
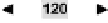








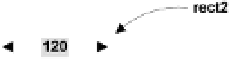
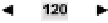




























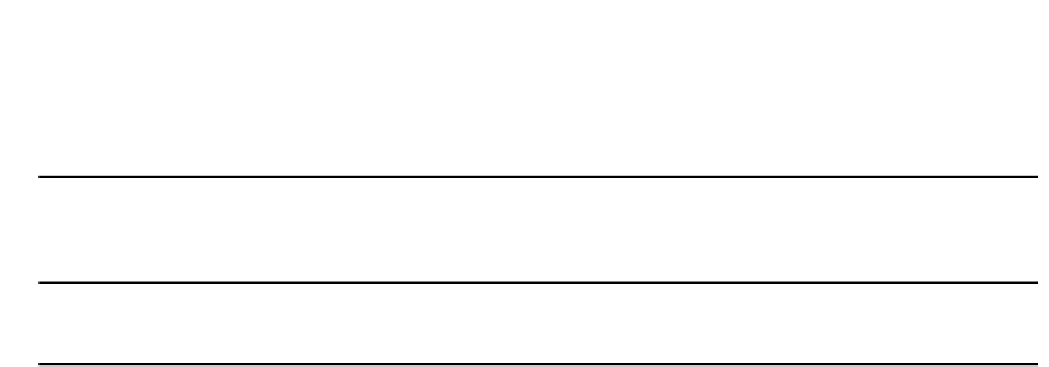