Java Reference
In-Depth Information
Events are not limited to window-based applications - they are a quite general
concept. Most programs that control or monitor things are event-driven. Any
occurrence external to a program such as a switch closing, or a preset temperature
being reached, can be registered as an event. In Java you can even create events
within your program to signal some other part of the code that something noteworthy
has happened. However, we're going to concentrate on the kinds of events that occur
when you interact as a user with a program.
The Event-handling Process
To manage the user's interaction with the components that make up the GUI for a program, we must
understand how events are handled in Java. To get an idea of how this works, let's consider a specific
example. Don't worry too much about the class names and other details here. Just try to get a feel for
how things connect together.
Suppose the user clicks a button in the GUI for your program. The button is the
source
of this event.
The event generated as a result of the mouse click is associated with the
JButton
object in your
program that represents the button on the screen. An event always has a source object - in this case the
JButton
object. When the button is clicked, it will create a new object that represents and identifies
this event - in this case an object of type
ActionEvent
. This object will contain information about the
event and its source. Any event that is passed to a Java program will be represented by a particular
event object - and this object will be passed as an argument to the method that is to handle the event.
OK
The listener connects to
the event source
public class My Button Handler
implements ActionListenes{
// class Members...
// Constuctor...
...
button.addActionListener(this),
...
void actionPerformed(ActionEvent)}
// Handle the Event...
by calling
method for the source
object.
addActionListener()
ActionEvent
object
Passed to
}
}
The event object corresponding to the button click will be passed to any
listener
object that has
previously registered an interest in this kind of event - a listener object being simply an object that
listens for particular events. A listener is also called a
target
for an event. Here, 'passing the event to the
listener' just means the event source calling a particular method in the listener object and passing the
event object to it as an argument. A listener object can listen for events for a particular object - just a
single button for instance, or it can listen for events for several different objects - a group of menu items
for example. Which approach you take depends on the context, and which is most convenient from a
programming point of view. Your programs will often involve both.

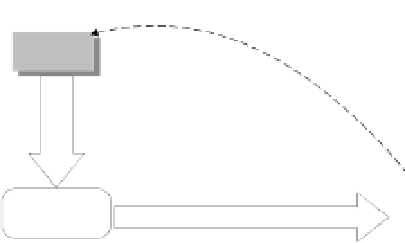


