Java Reference
In-Depth Information
Try It Out
- Playing with Point Objects
Try the following code:
import java.awt.Point;
public class PlayingPoints
public static void main(String[] args) {
Point aPoint = new Point(); // Initialize to 0,0
Point bPoint = new Point(50,25);
Point cPoint = new Point(bPoint);
System.out.println("aPoint is located at: " + aPoint);
aPoint.move(100,50); // Change to position 100,50
bPoint.x = 110;
bPoint.y = 70;
aPoint.translate(10,20); // Move by 10 in x and 20 in y
System.out.println("aPoint is now at: " + aPoint);
if(aPoint.equals(bPoint))
System.out.println("aPoint and bPoint are at the same location.");
}
}
If you run the program you should see:
aPoint is located at: java.awt.Point[x=0,y=0]
aPoint is now at: java.awt.Point[x=110,y=70]
aPoint and bPoint are at the same location.
How It Works
You can see the three constructors that the
Point
class provides in action in the first few lines. We then
manipulate the
Point
objects we've instantiated.
You can change a
Point
object to a new position with the
move()
method. Alternatively, you can use
the
setLocation()
method to set the values of the
x
and
y
members. The
setLocation()
method
does exactly the same as the
move()
method. It is included in the
Point
class for compatibility with
the
setLocation()
method for a component. For the same reason, there is also a
getLocation()
method in the
Point
class that returns a copy of the current
Point
object. As the example shows, you
can also translate a
Point
object by specified distances in the
x
and
y
directions using the
translate()
method.
Lastly, you can compare two
Point
objects using the
equals()
method. This compares the
x
and
y
coordinates of the two
Point
objects, and returns
true
if both are equal. The final output statement is
executed because the
Point
objects are equal.
Note that this is not the only class that represents points. We will see other classes that define points
when we discuss how to draw in a window.
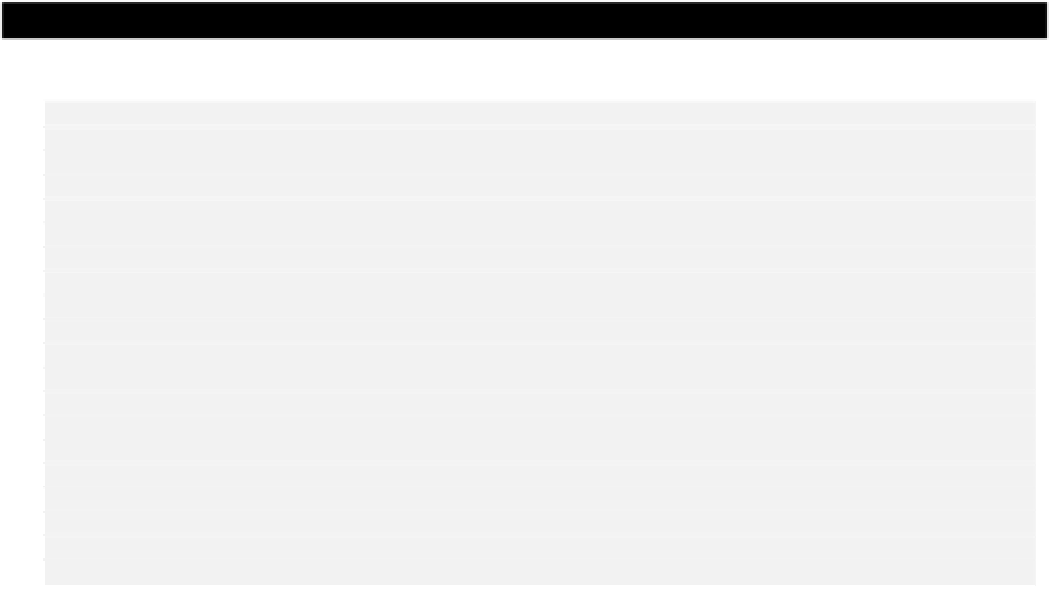







