Java Reference
In-Depth Information
You can display an application window simply by creating an object of type
JFrame
, calling a method
for the object to set the size of the window, and then calling a method to display the window. Let's try
that right away.
Try It Out
- Framing a Window
Here's the code:
import javax.swing.JFrame;
public class TryWindow {
// The window object
static JFrame aWindow = new JFrame("This is the Window Title");
public static void main(String[] args) {
int windowWidth = 400; // Window width in pixels
int windowHeight = 150; // Window height in pixels
aWindow.setBounds(50, 100, // Set position
windowWidth, windowHeight); // and size
aWindow.setDefaultCloseOperation(JFrame.EXIT
_
ON
_
CLOSE);
aWindow.setVisible(true); // Display the window
}
}
Under Microsoft Windows, the program will display the window shown:
Try resizing the window by dragging a border or a corner with the mouse. You can also try minimizing
the window by clicking on the icons to the right of the title bar. Everything should work OK so we are
getting quite a lot for so few lines of code. You can close the application by clicking on the
icon.
This example will terminate OK if you have entered the code correctly, however
errors could prevent this. If an application doesn't terminate properly for any reason
you will have to get the operating system to end the task. Under MS Windows,
switching to the DOS window and pressing
Ctrl+C
will do it.
How It Works
The
import
statement adds
JFrame
in the package
javax.swing
to our program. From now on
most of our programs will be using the components defined in this package. The object of type
JFrame
is created and stored as the initial value for the
static
data member of the class
TryWindow
, so it will
be created automatically when the
TryWindow
class is loaded. The argument to the constructor defines
the title to be displayed in the application window.
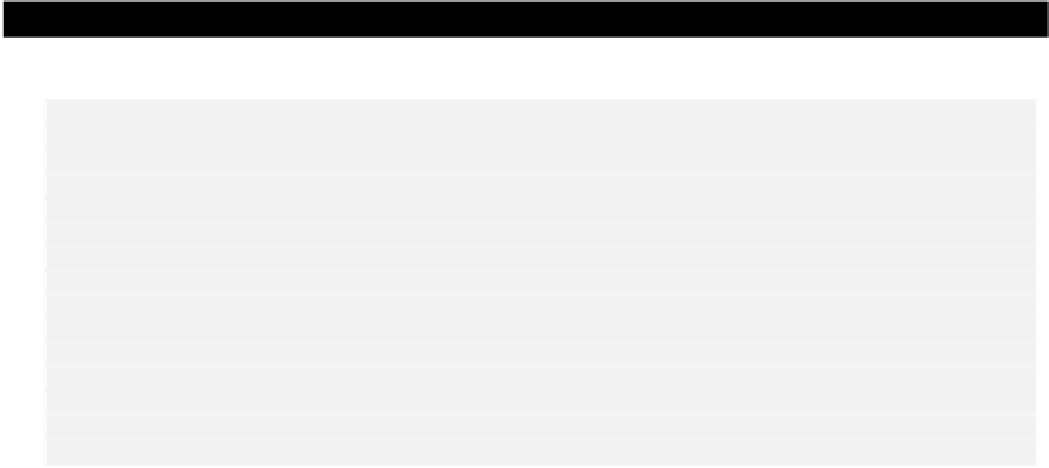
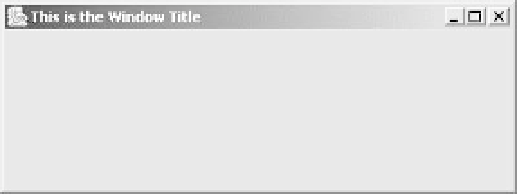
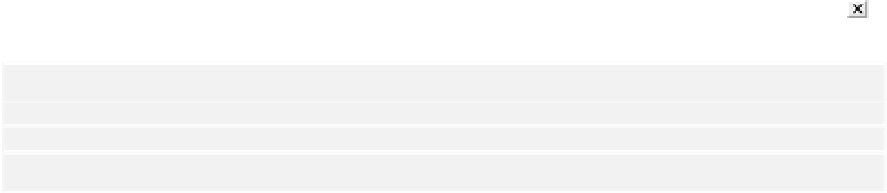













