Java Reference
In-Depth Information
These three processes that we have identified that run more or less independently of one another - one
to read the file, another to process the data, and a third to write the results - are called
threads
. Of
course, the first example at the top of the diagram has just one thread that does everything in sequence.
Every Java program has at least one thread. However, the three threads in the lower example aren't
completely independent of one another. After all, if they were, you might as well make them
independent programs. There are practical limitations too - the potential for overlapping these threads
will be dependent on the capabilities of your computer, and of your operating system. However, if you
can get some overlap in the execution of the threads, the program is going to run faster. There's no
magic in using threads, though. Your computer has only a finite capacity for executing instructions, and
if you have many threads running you may in fact increase the overall execution time because of the
overhead implicit in managing the switching of control between threads.
An important consideration when you have a single program running as multiple threads is that the
threads are unlikely to have identical execution times, and, if one thread is dependent on another, you
can't afford to have one overtaking the other - otherwise you'll have chaos. Before you can start
calculating in the example in the diagram, you need to be sure that the data block the calculation uses
has been read, and before you can write the output, you need to know that the calculation is complete.
This necessitates having some means for the threads to communicate with one another.
The way we have shown the threads executing in the previous diagram isn't the only way of organizing
the program. You could have three threads, each of which reads the file, calculates the results, and
writes the output, as shown here.
Multiple Threads
thread 1
read block 1
calculate 1
write 1
read block 4
calculate 4
thread 2
read block 2
calculate 2
write 2
read block 5
thread 3
read block 3
calculate 3
write 3
time
Now there's a different sort of contention between the threads. They are all competing to read the file
and write the results, so there needs to be some way of preventing one thread from getting at the input
file while another thread is already reading from it. The same goes for the output file. There's another
aspect of this arrangement that is different from the previous version. If one thread,
thread1
say, reads a
block,
block4
perhaps, that needs a lot of time to compute the results, another thread,
thread2
say, could
conceivably read a following block,
block5
maybe, and calculate and write the results for
block5
before
thread1
has written the results for
block4
. If you don't want the results appearing in a different sequence
from the input, you should do something about this. Before we delve into the intricacies of making sure
our threads don't get knotted, let's first look at how we create a thread.
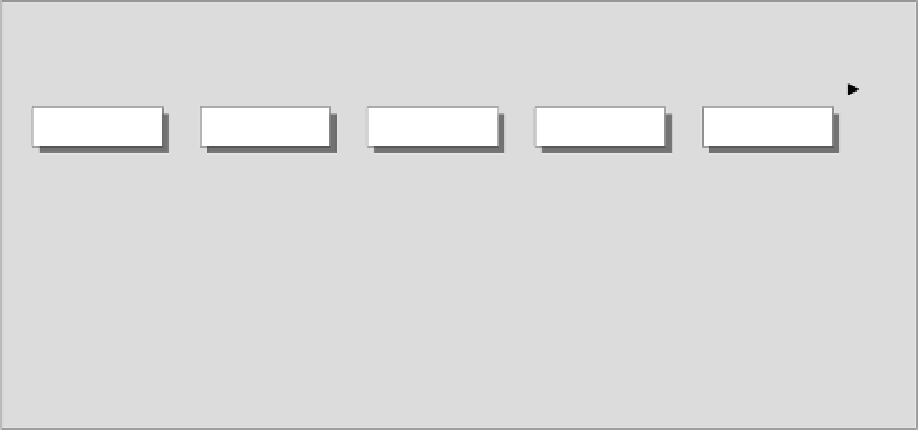

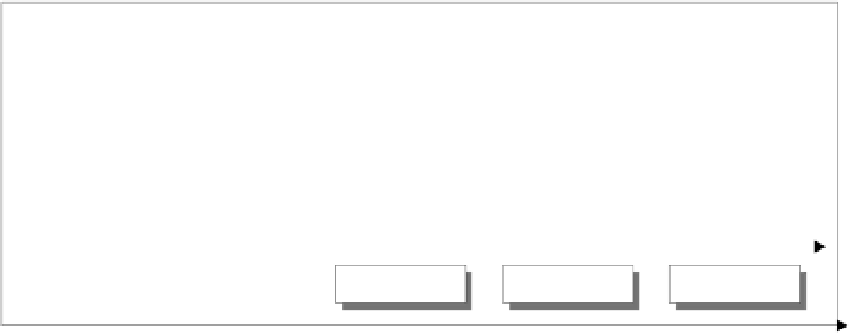






