Java Reference
In-Depth Information
With the aid of another
static
method from the
Collections
class it couldn't be easier. The
shuffle()
method in
Collections
shuffles the contents of any collection that implements the
List
interface, so we end up with a shuffled deck of
Card
objects. For those interested in the details of shuffling,
this
shuffle()
method randomly permutes the list by running backwards through its elements swapping
the current element with a randomly chosen element between the first and the current element. The time
taken to complete the operation is proportional to the number of elements in the list.
An overloaded version of the
shuffle()
method allows you to supply an object of type
Random
as
the second argument that is used for selecting elements at random while shuffling.
The final piece is a class that defines
main()
:
class TryDeal {
public static void main(String[] args) {
CardDeck deck = new CardDeck();
deck.shuffle();
Hand myHand = deck.dealHand(5);
Hand yourHand = deck.dealHand(5);
System.out.println("\nMy hand is" + myHand);
System.out.println("\nYour hand is" + yourHand);
}
}
I got the output:
My hand is 3D KD 7D 8C 7S
Your hand is 9C 6D 6C 3C AS
You will almost certainly get something different.
How It Works
The code for
main()
first creates a
CardDeck
object and calls its
shuffle()
method to randomize
the sequence of
Card
objects. It then creates two
Hand
objects of 5 cards by calling the
dealHand()
method. The output statements just display the hands that were dealt.
A
Stack
object is particularly suited to dealing cards, as we want to remove each card from the deck as
it is dealt and this is done automatically by the
pop()
method that retrieves an object. When we need
to go through the objects in a
Stack
collection without removing them, we can use an iterator as we
did in the
toString()
method in the
Hand
class. Of course, since the
Stack
class is derived from
Vector
, all the
Vector
class methods are available too, when you need them.
I think you'll agree that using a stack is very simple. A stack is a powerful tool in many different
contexts. A stack is often applied in applications that involve syntactical analysis, such as compilers and
interpreters - including those for Java.






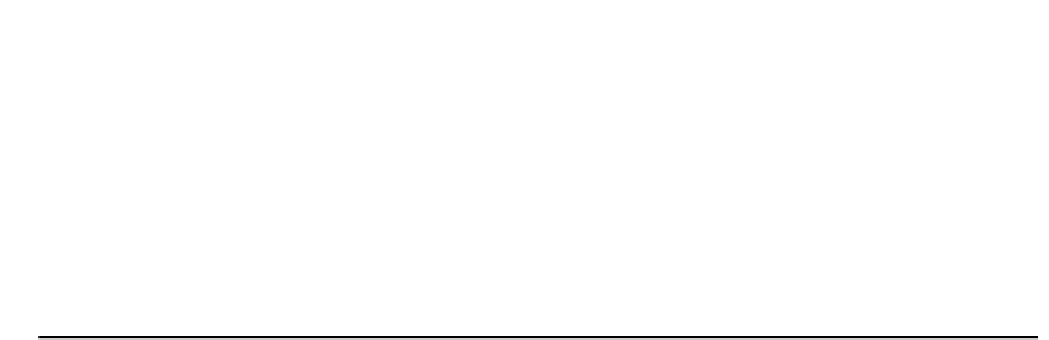