Java Reference
In-Depth Information
We need a constructor that ensures that the suit and face value of a card are legal values. We can
implement the constructor like this:
class Card {
public Card(int value, int suit) throws IllegalArgumentException {
if(value >= ACE && value <= KING)
this.value = value;
else
throw new IllegalArgumentException("Invalid card value");
if(suit >= HEARTS && suit <= SPADES)
this.suit = suit;
else
throw new IllegalArgumentException("Invalid suit");
}
// Other members as before...
}
To deal with invalid arguments to the constructor, we just throw an exception of the standard type,
IllegalArgumentException
, that we create with a suitable message.
We will undoubtedly need to display a card so we will need a
String
representation of a
Card
object.
The
toString()
method will do this for us:
class Card {
public String toString() {
String cardStr;
switch(value) {
case ACE: cardStr = "A";
break;
case JACK: cardStr = "J";
break;
case QUEEN: cardStr = "Q";
break;
case KING: cardStr = "K";
break;
default: cardStr = Integer.toString(value);
}
switch(suit) {
case CLUBS: cardStr += "C";
break;
case DIAMONDS: cardStr += "D";
break;
case HEARTS: cardStr += "H";
break;
case SPADES: cardStr += "S";
break;
default:
assert false; // We should never get to here
}

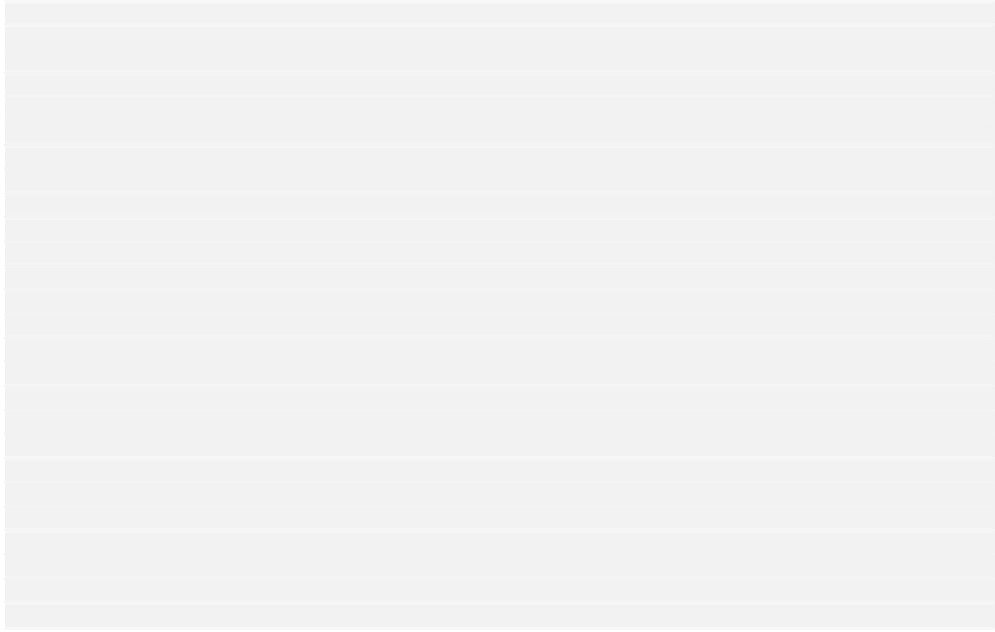





