Java Reference
In-Depth Information
Enter first name or ! to end:
!
You added 3 people to the cast.
Roy Rogers
Marilyn Monroe
Robert de Niro
How It Works
Here we'll be assembling an all-star cast for a new blockbuster. The method
main()
creates a
Person
variable, which will be used as a temporary store for an actor or actress, and a
Crowd
object
filmCast
, to hold the entire cast.
The
for
loop uses the
readPerson()
method to obtain the necessary information from the keyboard
and create a
Person
object. If
!
or
"!"
is entered from the keyboard,
readPerson()
will return
null
and this will end the input process for cast members.
We then output the members of the cast by obtaining an
Iterator
. As you see, this makes the code
for listing the members of the cast very simple. Instead of an iterator we could also have used the
get()
method that we implemented in
Crowd
to retrieve the actors:
for(int i = 0 ; i<filmCast.size() ; i++)
System.out.println(filmCast.get());
Sorting
The output from the last example appears in the sequence in which you enter it. If we want to be
socially correct, say, in the creation of a cast list, we should arrange them in alphabetical order. We
could write our own method to sort
Person
objects in the
Crowd
object, but it will be a lot less trouble
to take advantage of another feature of the
java.util
package, the
Collections
class - not to be
confused with the
Collection
interface. The
Collections
class defines a variety of handy static
methods that you can apply to collections, and one of them happens to be a
sort()
method.
The
sort()
method will only sort lists, that is, collections that implement the
List
interface. Obviously
there also has to be some way for the
sort()
method to determine the order of objects from the list that it is
sorting - in our case,
Person
objects. The most suitable way to do this for
Person
objects is to implement
the
Comparable
interface for the class. The
Comparable
interface only declares one method,
compareTo()
. We saw this method in the
String
class so you know it returns -1, 0, or +1 depending on
whether the current object is less than, equal to, or greater than the argument passed to the method. If the
Comparable
interface is implemented for the class, we just pass the collection object as an argument to the
sort()
method. The collection is sorted in place so there is no return value.
We can implement the
Comparable
interface very easily for our
Person
class, as follows:
public class Person implements Comparable {
// Constructor
public Person(String firstName, String surname) {












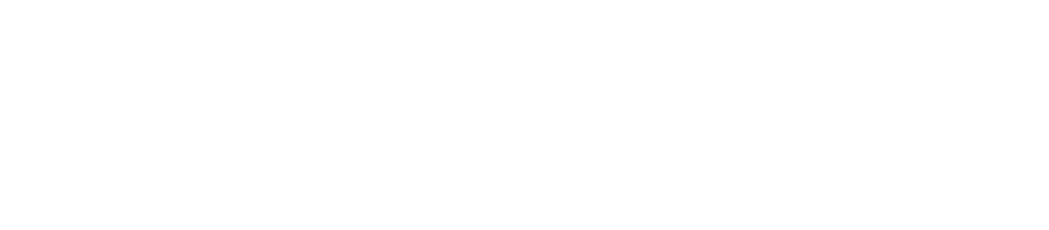