Java Reference
In-Depth Information
With all these ways of removing elements from a
Vector
, there's a lot of potential for ending up with
an empty
Vector
. It's often handy to know whether a
Vector
contains elements or not, particularly if
there's been a lot of adding and deleting of elements. You can check whether or not a
Vector
contains
elements by using the
isEmpty()
method. This returns
true
if a
Vector
object has zero
size
, and
false
otherwise.
Note that a
Vector
may contain only
null
references, but this doesn't mean the
size()
will be
zero or that
isEmpty()
will return
true
. To empty a
Vector
object you must actually remove
the elements, not just set the elements to
null
.
Searching a Vector
You can get the index position of an object stored in a
Vector
by passing the object as an argument to
the method
indexOf()
. For example, the statement
int position = transactions.indexOf(aTransaction);
will search the
Vector
from the beginning for the object
aTransaction
using the
equals()
method
for the argument, so your class needs to have a proper implementation of
equals()
for this to work.
The variable
position
will contain either the index of the first reference to the object in
transactions
, or -1 if the object isn't found.
You have another version of the method
indexOf()
available that accepts a second argument that is
the index position where the search for the object should begin. The main use for this arises when an
object can be referenced more than once in a
Vector
. You can use the method in this situation to
recover all occurrences of any particular object, as follows:
int position = -1; // Search starting index
while(++position<transactions.size()) { // Search with a valid index
if(position = transactions.indexOf(aTransaction, position))<0) // Find next
break;
// Code to process the object in some way...
}
The
while
loop will continue as long as the method
indexOf()
returns a valid index value and the
index doesn't get incremented beyond the end of the
Vector
.
position =
transactions.indexOf(aTransaction,position)
Use to start next search
Starts at 0
index of object found
aTransaction
aTransaction
Increment
++position

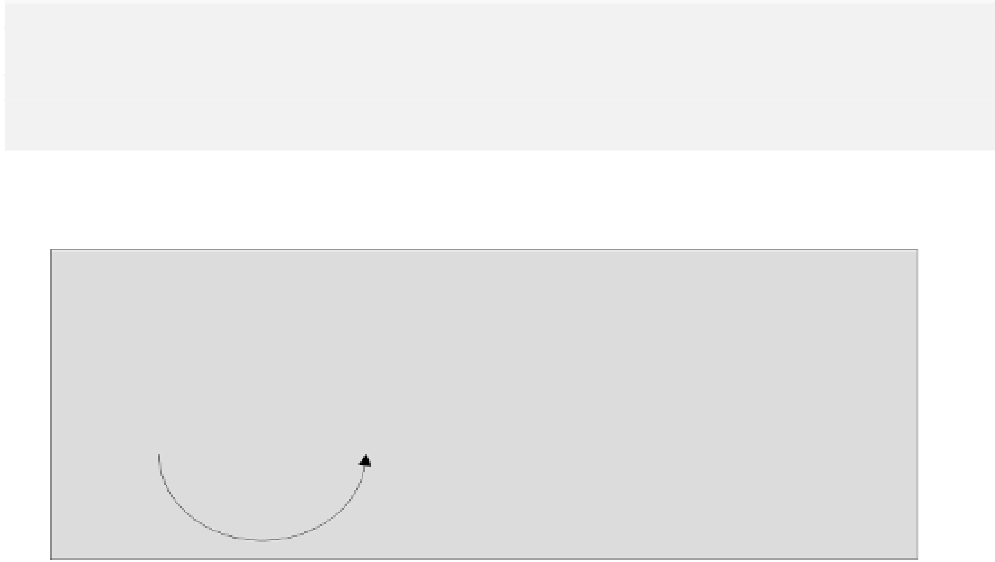












