Java Reference
In-Depth Information
The
static
sleep()
method in the
Thread
class causes the current thread to sleep for the number
of milliseconds specified by the argument. While our current thread is sleeping other threads can
execute, so whoever has a lock on our file has a chance to release it.
Here's the code for the complete example:
import java.io.*;
import java.nio.*;
import java.nio.channels.*; // For FileChannel and FileLock
public class LockingPrimesRead {
public static void main(String[] args)
File aFile = new File("C:/Beg Java Stuff/primes.bin");
FileInputStream inFile = null;
try {
inFile = new FileInputStream(aFile);
} catch(FileNotFoundException e) {
e.printStackTrace(System.err);
System.exit(1);
}
FileChannel inChannel = inFile.getChannel();
final int PRIMECOUNT = 6;
ByteBuffer buf = ByteBuffer.allocate(8*PRIMECOUNT);
long[] primes = new long[PRIMECOUNT];
try {
int primesRead = 0;
FileLock inLock = null;
while(true) {
int tryLockCount = 0;
// Get a lock on the file region we want to read
while(true) {
inLock = inChannel.tryLock(inChannel.position(),
buf.remaining(),
false);
if(inLock == null) {
if(++tryLockCount <100)
{
try {
Thread.sleep(200); // Wait for 200 milliseconds
} catch(InterruptedException e) {
e.printStackTrace(System.err);
}
continue; // Continue with next loop iteration
} else {
System.out.println("Failed to acquire lock after "
+ tryLockCount+" tries."+ " Terminating...");
System.exit(1);
} else {
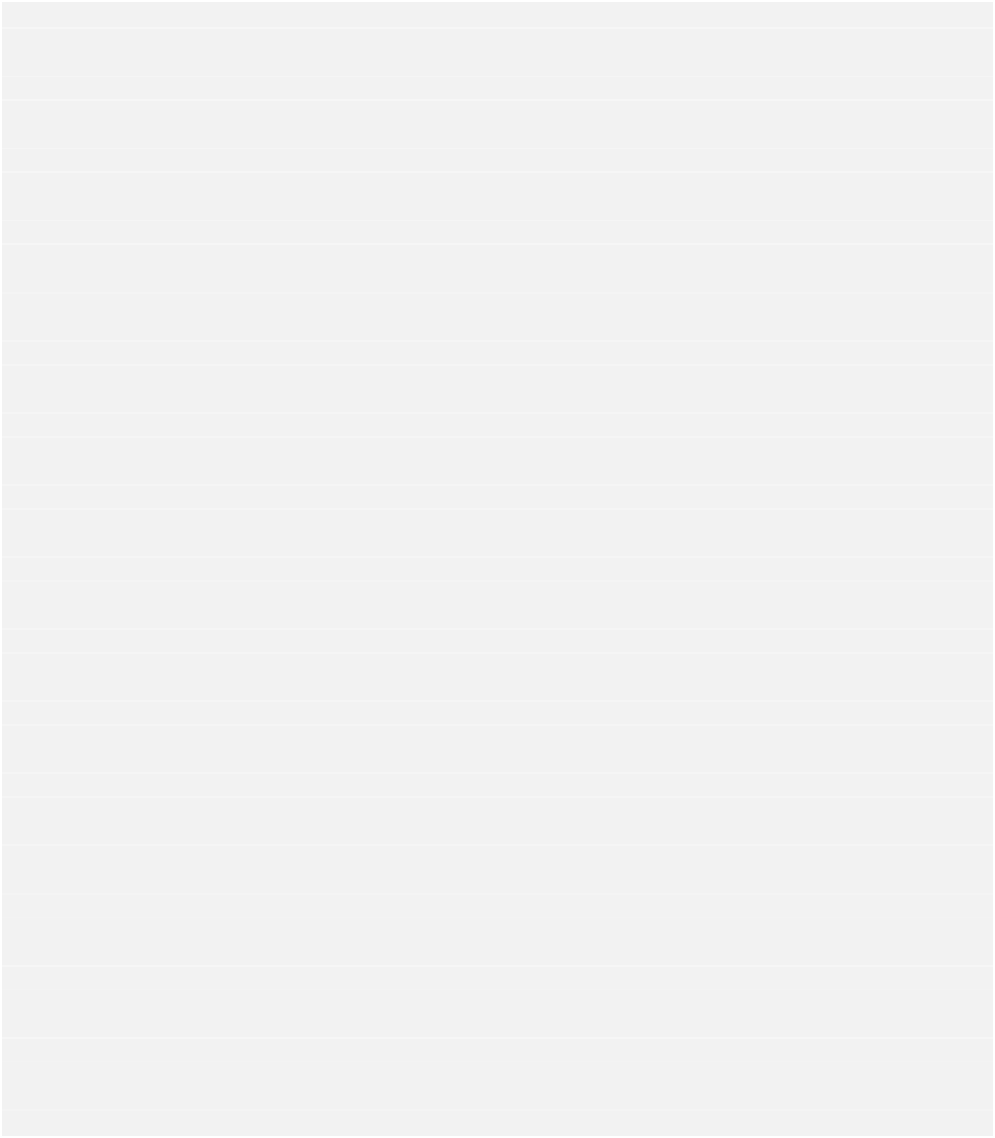



