Java Reference
In-Depth Information
force()
If the buffer was mapped in
MAP
_
RW
mode this method forces any changes
made to the buffer's contents to be written to the file and returns a reference to
the buffer. For buffers created with
MAP
_
RO
or
MAP
_
COW
mode this method has
no effect.
load()
Loads the contents of the buffer into memory and returns a reference to the
buffer.
isLoaded()
Returns true if this buffer's contents is available in physical memory and false
otherwise.
Using a memory-mapped file through a
MappedByteBuffer
is simplicity itself, so let's try it.
Try It Out - Using a Memory-Mapped File
We will access and modify the
primes.bin
file using a
MappedByteBuffer
. Here's the code:
import java.io.*;
import java.nio.*;
import java.nio.channels.FileChannel;
public class MemoryMappedFile {
public static void main(String[] args) {
File aFile = new File("C:/Beg Java Stuff/primes.bin");
RandomAccessFile ioFile = null;
try {
ioFile = new RandomAccessFile(aFile,"rw");
} catch(FileNotFoundException e) {
e.printStackTrace(System.err);
System.exit(1);
}
FileChannel ioChannel = ioFile.getChannel();
final int PRIMESREQUIRED = 10;
long[] primes = new long[PRIMESREQUIRED];
int index = 0; // Position for a prime in the file
final long REPLACEMENT = 999999L; // Replacement for a selected prime
try {
final int PRIMECOUNT = (int)ioChannel.size()/8;
MappedByteBuffer buf =
ioChannel.map(ioChannel.MAP
_
RW, 0L, ioChannel.size()).load();
for(int i = 0 ; i<PRIMESREQUIRED ; i++) {
index = 8*(int)(PRIMECOUNT*Math.random());
primes[i] = buf.getLong(index);
buf.putLong(index, REPLACEMENT );
}
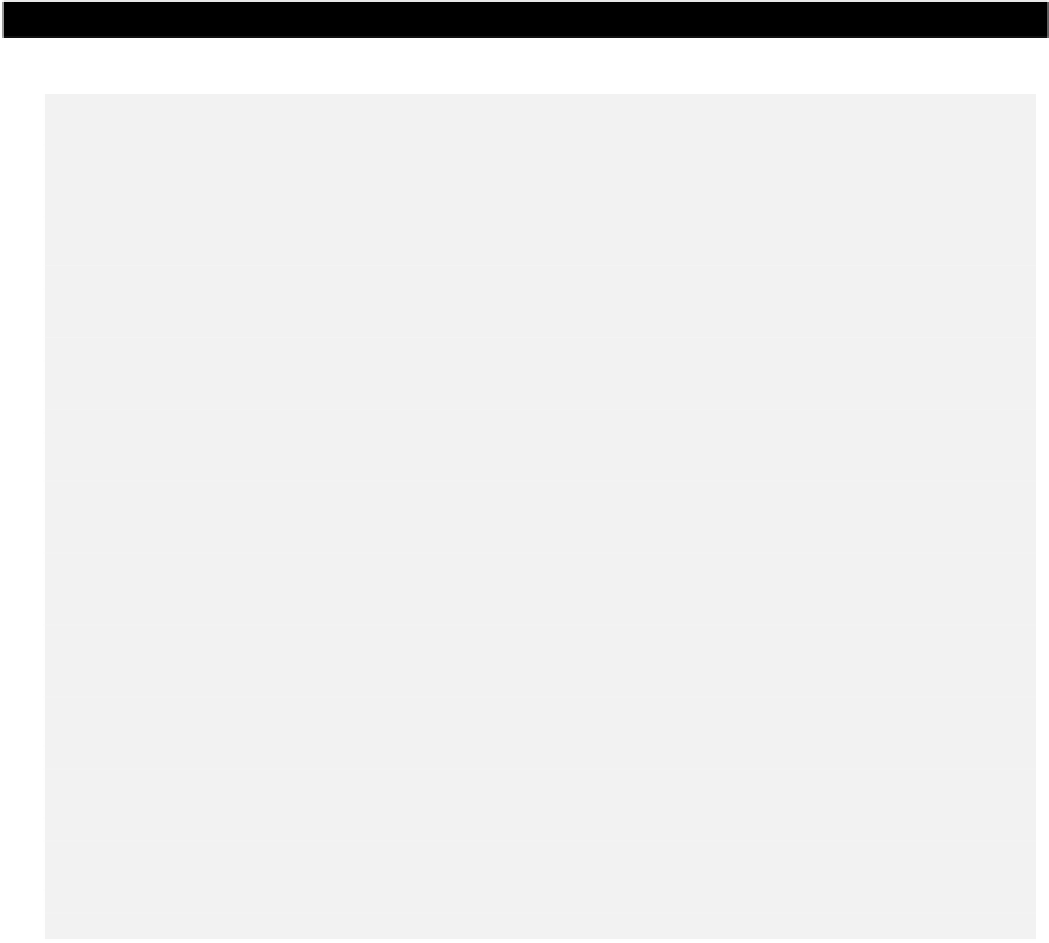








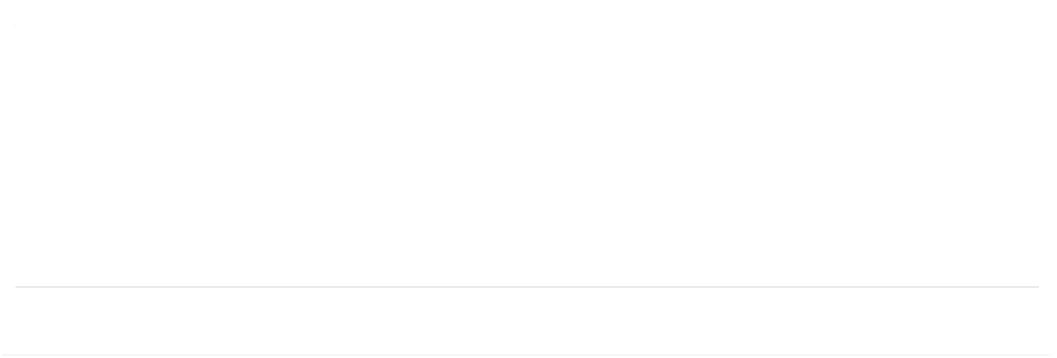