Java Reference
In-Depth Information
There are two constructors available for creating
RandomAccessFile
objects, and both require two
arguments. For one constructor, the first argument is a
File
object that identifies the file path, and the
second is a
String
object that specifies the access mode. The other constructor offer the alternative of
using a
String
object as the first argument specifying the file path, with the second argument defining
the access mode as before.
The access mode can be
"r"
, which indicates that you just want to read the file, or it can be
"rw"
,
which indicates that you want to open the file to allow both read and write operations. If you specify the
mode as anything else, the constructor will throw an
IllegalArgumentException
.
To create a
RandomAccessFile
object, you could write:
File myPrimes = new File("c:/Beg Java Stuff/primes.bin");
RandomAccessFile primesFile = null;
try {
primesFile = new RandomAccessFile(myPrimes, "rw");
} catch(FileNotFoundException e) {
e.printStackTrace(System.err);
assert false;
}
This will create the random access file object,
primesFile
, corresponding to the physical file,
primes.bin
, and will open it for both reading and writing.
If the file does not exist when you specify
"rw"
as the mode, the file will be created automatically as
long as the parent directory exists. Of course, there is the implicit assumption that you intend to write to
the file before you try to read it. If you specify the mode as
"r"
, the file must already exist. If it doesn't,
the constructor will throw a
FileNotFoundException
. The same exception will be thrown if the file
exists but cannot be opened for some reason. Like the file stream class constructors, a
RandomAccessFile
constructor can throw a
SecurityException
if a security manager exists and
access to the file is denied.
You obtain the
FileChannel
object from a
RandomAccessFile
object in the same way as for the
file stream objects we have been working with:
FileChannel ioChannel = primesFile.getChannel();
You can now use this channel to read and write the file sequentially or at random. It should be a trivial
exercise for you to modify the previous example to use a channel from a
RandomAccessFile
object
instead of the channels for the two file stream objects.
If you check the documentation for the
RandomAccessFile
class, you will find that it too records the
file position and it describes it as a file-pointer. It also defines the
getFilePointer()
method for
obtaining the current value of the file-pointer and the
seek()
method that alters it. The file-pointer for
a
RandomAccessFile
object is identical to the file position recorded by its channel, and changes to
one are immediately reflected in the other.






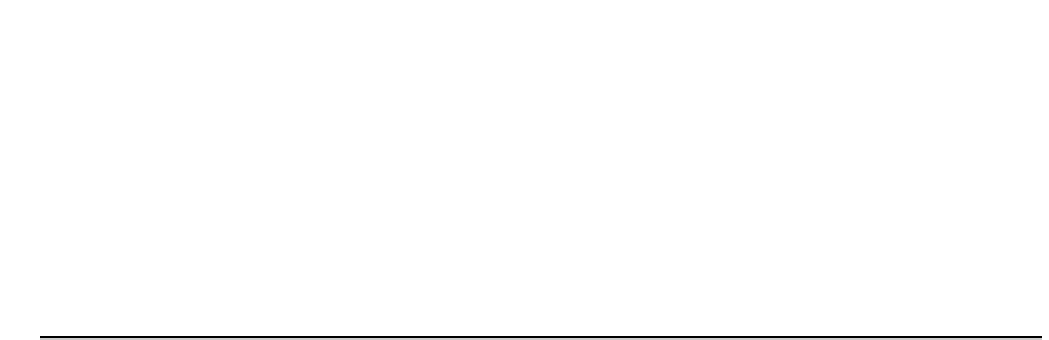