Java Reference
In-Depth Information
ByteBuffer buf = ByteBuffer.allocateDirect (1024);
buf.position(buf.limit()); // Set the position for the loop operation
int strLength = 0; // Stores the string length
byte[] strChars = null; // Array for string
while(true) {
if(buf.remaining() < 8) { // Verify enough bytes for string length
if(replenish(inChannel, buf) == -1) // If not, replenish the buffer
break; // but exit loop on EOF
else
buf.flip();
}
strLength = (int)buf.getDouble();
// Verify enough bytes for complete string
if(buf.remaining()<strLength) {
if(replenish(inChannel, buf) == -1) // If not, replenish the buffer
assert false; // and we should never arrive here
else
buf.flip();
}
strChars = new byte[strLength];
buf.get(strChars);
if(buf.remaining()<8) { // Verify enough bytes for prime value
if(replenish(inChannel, buf) == -1) // If not, replenish the buffer
assert false; // and we should never arrive here
else
buf.flip();
}
System.out.println("String length: " + strChars.length + " String: " +
new String(strChars) + " Binary value: " +
buf.getLong());
}
System.out.println("\nEOF reached.");
inFile.close(); // Close the file and the channel
} catch(IOException e) {
e.printStackTrace(System.err);
System.exit(1);
}
System.exit(0);
}
private static int replenish(FileChannel channel, ByteBuffer buf)
throws IOException {
// Number of bytes left in file
long bytesLeft = channel.size() - channel.position();
if(bytesLeft == 0L) // If there are none
return -1; // we have reached the end
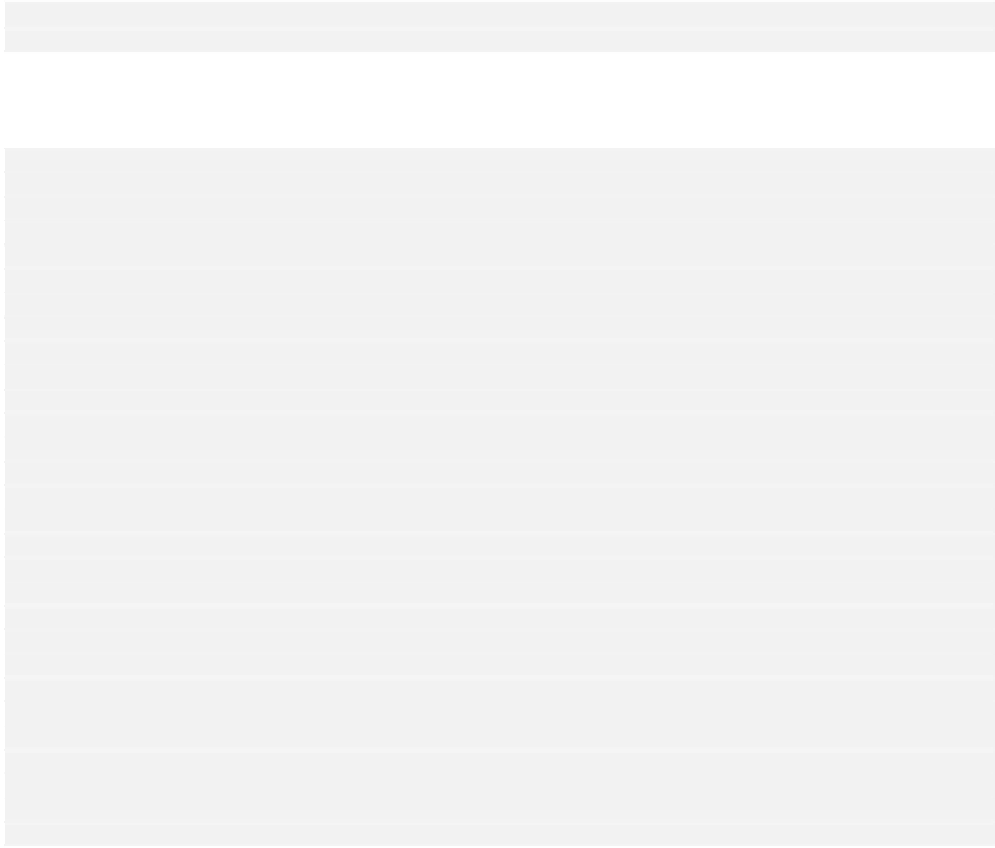



