Java Reference
In-Depth Information
Of course, with small buffers and limited amounts of data being read, using an indirect buffer doesn't
add much overhead. With large buffers and lots of data, it can make a significant difference though. In
this case, you can use the
allocateDirect()
method in the
ByteBuffer
class to allocate a
direct
buffer
. The JVM will try to make sure that the native I/O operation makes use of the direct buffer, thus
avoiding the overhead of the data copying process. The allocation and de-allocation of a direct buffer
carries its own overhead, which may outweigh any advantages gained if the buffer size and data
volumes are small.
You can test for a direct buffer by calling its
isDirect()
method. This will return
true
if it is a direct
buffer and
false
otherwise.
You could try this out by making a small change to the previous example. Just replace the statement:
ByteBuffer buf = ByteBuffer.allocate(2 * maxLength + 4);
with the following two statements:
ByteBuffer buf = ByteBuffer.allocateDirect(2 * maxLength + 4);
System.out.println("Buffer is "+ (buf.isDirect()?"":"not")+"direct.");
This will output a line telling you whether the program is working with a direct buffer or not. It will
produce the following output:
Buffer is direct.
Proverbs written to file.
Writing Numerical Data to a File
Let's see how we could set up our primes-generating program from Chapter 4 to write primes to a file
instead of outputting them. We will base the new code on the
MorePrimes
version of the program.
Ideally, we could add a command-line argument to specify how many primes we want. This is not too
difficult. Here's how the code will start off:
public class PrimesToFile {
public static void main(String[] args) {
int primesRequired = 100; // Default prime count
if (args.length > 0) {
try {
primesRequired = Integer.valueOf(args[0]).intValue();
} catch (NumberFormatException e) {
System.out.println("Prime count value invalid. Using default of "
+ primesRequired);
}
// Code to generate the primes...
// Code to write the file...
}
}
}

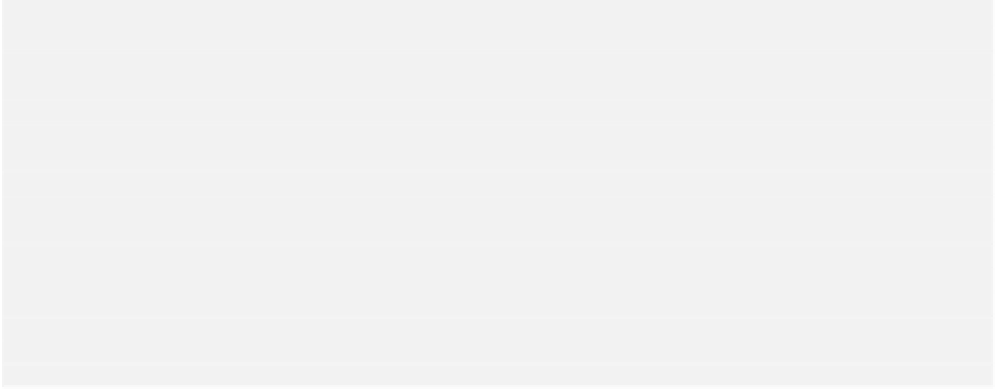









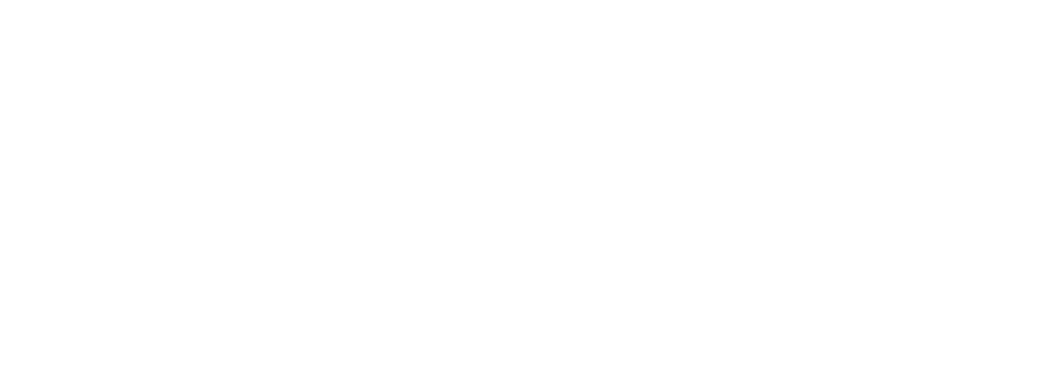