Java Reference
In-Depth Information
Exception
Description
ClosedChannelException
Thrown if the channel is closed.
AsynchronousCloseException
Thrown if another thread closes the channel
while the write operation is in progress.
ClosedByInterruptException
Thrown if another thread interrupts the current
thread while the write operation is in progress.
IOException
If any other I/O error occurs.
You might use this method in a sequence of writes to update a particular part of the file without
disrupting the primary sequence of write operations. For example, you might record a count of the
number of records in a file at the beginning. As you add new records to the file, you could update the
count at the beginning of the file without changing the file position recorded by the channel, which
would be pointing to the end of the file where new data is to be written.
You can find out what the current file position is by calling the
position()
method for the
FileChannel
object. This returns the position as type
long
rather than type
int
since it could
conceivably be a large file with a lot more than two billion bytes in it. You can also set the file position
by calling a position method for the
FileChannel
object, with an argument of type
long
specifying a
new position. For instance, if we have a reference to a file channel stored in a variable
outputChannel
, we could alter the file position with the statements:
try {
outputChannel.position(fileChannel.position() - 100);
} catch (IOException e) {
e.printStackTrace(System.err);
}
This moves the current file position back by 100 bytes. This could be because we have written 100 bytes
to the file and want to reset the position so we can rewrite it. The method must be in a
try
block
because it can throw an exception of type
IOException
if an I/O error occurs.
You can set the file position beyond the end of the file. If you then write to the file, the bytes between
the previous end of the file and the new position will contain junk values. If you try to read from a
position beyond the end of the file, an end-of-file condition will be returned immediately.
When you are finished with writing a file you should close it by calling the
close()
method for the file
stream object. This will close the file and the file channel. A
FileChannel
object defines its own
close()
method that will close the channel but not the file. It's time to start exercising your disk drive.
Let's try an example.
Try It Out - Using a Channel to Write a String to a File
We will write the string
"Garbage
in,
garbage
out\n"
to a file,
charData.txt
, that we will
create in the directory
Beg
Java
Stuff
on drive
C:
. If you want to write to a different drive and/or
directory, just change the program accordingly. Here is the code:

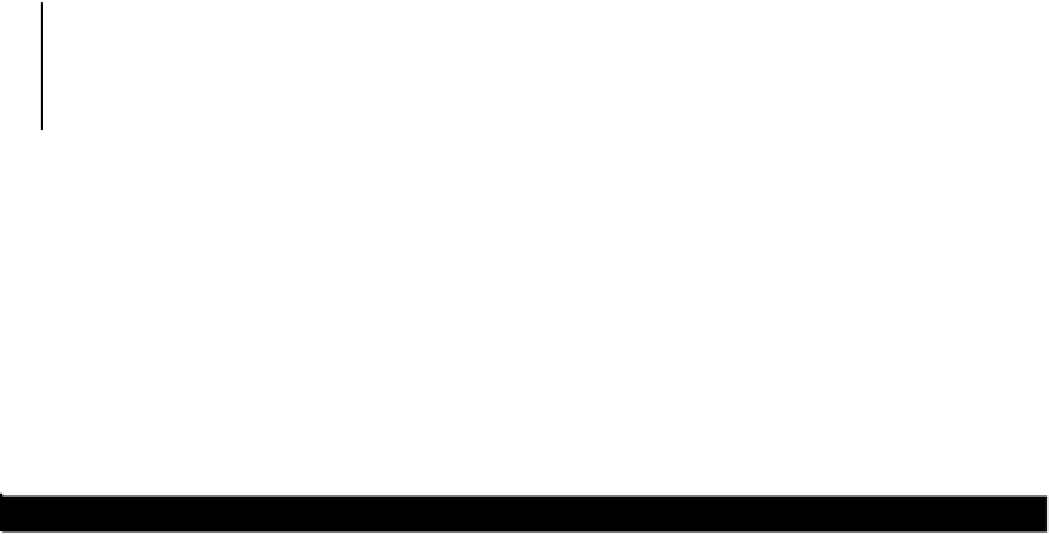








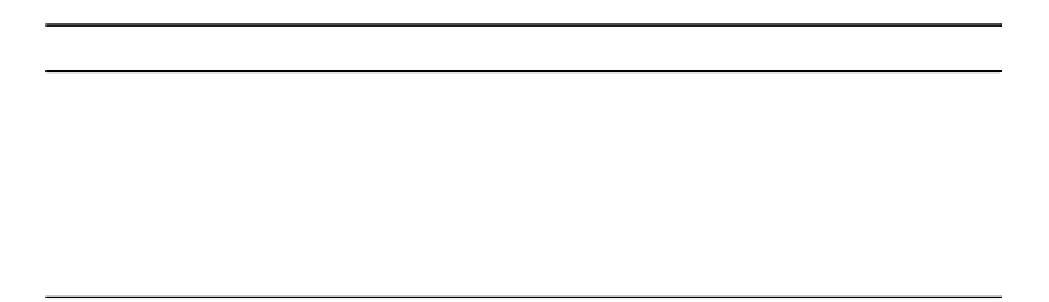