Java Reference
In-Depth Information
The
listFiles()
method returns a
File
array, with each element of the array representing a
member of the directory, which could be a subdirectory or a file. We store the reference to the array
returned by the method in our array variable
contents
. After outputting a heading, we check that the
array is not
null
. We then list the contents of the directory in the
for
loop. We use the
isDirectory()
method to determine whether each item is a file or a directory, and create the output
accordingly. We could just as easily have used the
isFile()
method here. The
lastModified()
method returns a
long
value that represents the time when the file was last modified in milliseconds
since midnight on January 1st 1970. To get this to a more readable form, we use the value to create a
Date
object, and the
toString()
method for the class returns what you see in the output. The
Date
class is defined in the
java.util
package. (See Chapter 13.) We have imported this into the program
file but we could just as well use the fully qualified class name,
java.util.Date
, instead. If the
contents
array is
null
, we just output a message. You could easily add code to output the length of
each file here, if you want.
Note that there is a standard system property with the name
java.home
that identifies the directory
that is the root directory of the Java runtime environment. If you have installed the SDK (rather than
just a JRE), this will be the
jre
subdirectory to the SDK subdirectory, which on my system is
C:/SDK1.4
. In this case the value of
java.home
will be
"C:/sdk1.4/jre"
. You could therefore
use this to refer to the file in the previous example in a system-independent way. If you create a
File
object from the value of the
java.home
property, calling its
getParent()
method will return the
parent directory as a
String
object. This will be the SDK home directory so you could use this as the
base directory to access the source files for the class libraries, like this:
File myDir = new File(new File(System.getProperty("java.home")).getParent(),
"src" + File.separator+"java" + File.separator+"io");
As long as the JRE in effect is the one installed as part of the SDK, we have a system-independent way
of accessing the source files for the library classes.
Filtering a File List
The
list()
and
listFiles()
methods are overloaded with versions that accept an argument used to filter
a file list. This enables you to get a list of those files with a given extension, or with names that start with a
particular sequence of characters. For example, you could ask for all files starting with the letter 'T', which
might return the two files we created above: "
TryFile.java
" and "
TryFile2.java
". The argument that
you pass to the
list()
method must be a variable of type
FilenameFilter
whereas the
listFiles()
method is overloaded with versions to accept arguments of type
FilenameFilter
or
FileFilter
. Both
FilenameFilter
and
FileFilter
are interfaces that contain the abstract method
accept()
. The
FilenameFilter
interface is defined in the
java.io
package as:
public interface FilenameFilter {
public abstract boolean accept(File directory, String filename);
}
The
FileFilter
interface, also defined in
java.io
, is very similar:
public interface FileFilter {
public abstract boolean accept(File pathname);
}

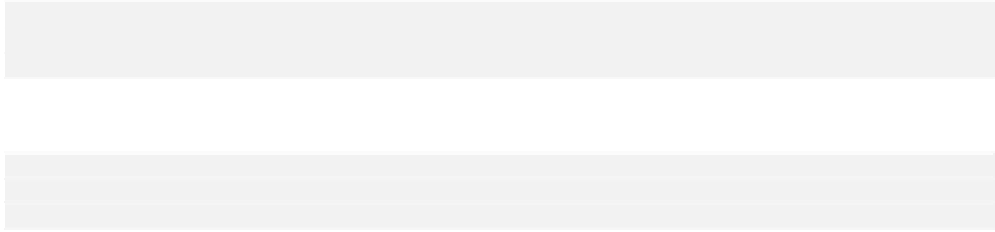








