Java Reference
In-Depth Information
Calling
getParent()
for
dataFile
here will return
null
. However, we can create a new
File
object encapsulating the absolute path:
dataFile = dataFile.getAbsoluteFile();
Now
dataFile
refers to a new object that encapsulates the absolute path to "
output.txt
", so calling
getParent()
will return the path to the parent directory - which will correspond to the value of the
user.dir
system property in this case.
Note that all operations involving the access of files on the local machine can throw a
SecurityException
if access is not authorized - in an applet for instance. This is
the case with all of the methods here. However, for a
SecurityException
to be
thrown, a security manager must exist on the local machine, but by default a Java
application has no security manager. An applet, on the other hand, always has a
security manager, by default. A detailed discussion of Java security is beyond the
scope of this topic. A comprehensive discussion of this topic is provided by the topic
"Professional Java Security" by Jess Garms and Daniel Somerfield, published by
Wrox Press, ISBN 1861004257
.
To see how some of these methods go together, we can try a simple example.
Try It Out - Testing for a File
Try the following source code. Don't forget the
import
statement for the
java.io
package, since the
example won't compile without it. The source code is in a zip called
src.jar
, and can be found in the
home directory for the JDK. If you haven't installed the Java source code on your system, you could try
the example with the file that contains the source code for the example itself -
TryFile.java
:
In all the examples in this chapter, you may need to specify substitute paths to suit
your environment.
import java.io.File;
public class TryFile {
public static void main(String[] args) {
// Create an object that is a directory
File myDir = new File("C:/j2sdk1.4.0/src/java/io");
System.out.println(myDir + (myDir.isDirectory() ? " is" : " is not")
+ " a directory.");
// Create an object that is a file
File myFile = new File(myDir, "File.java");
System.out.println(myFile + (myFile.exists() ? " does" : " does not")
+ " exist");
System.out.println(myFile + (myFile.isFile() ? " is" : " is not")
+ " a file.");
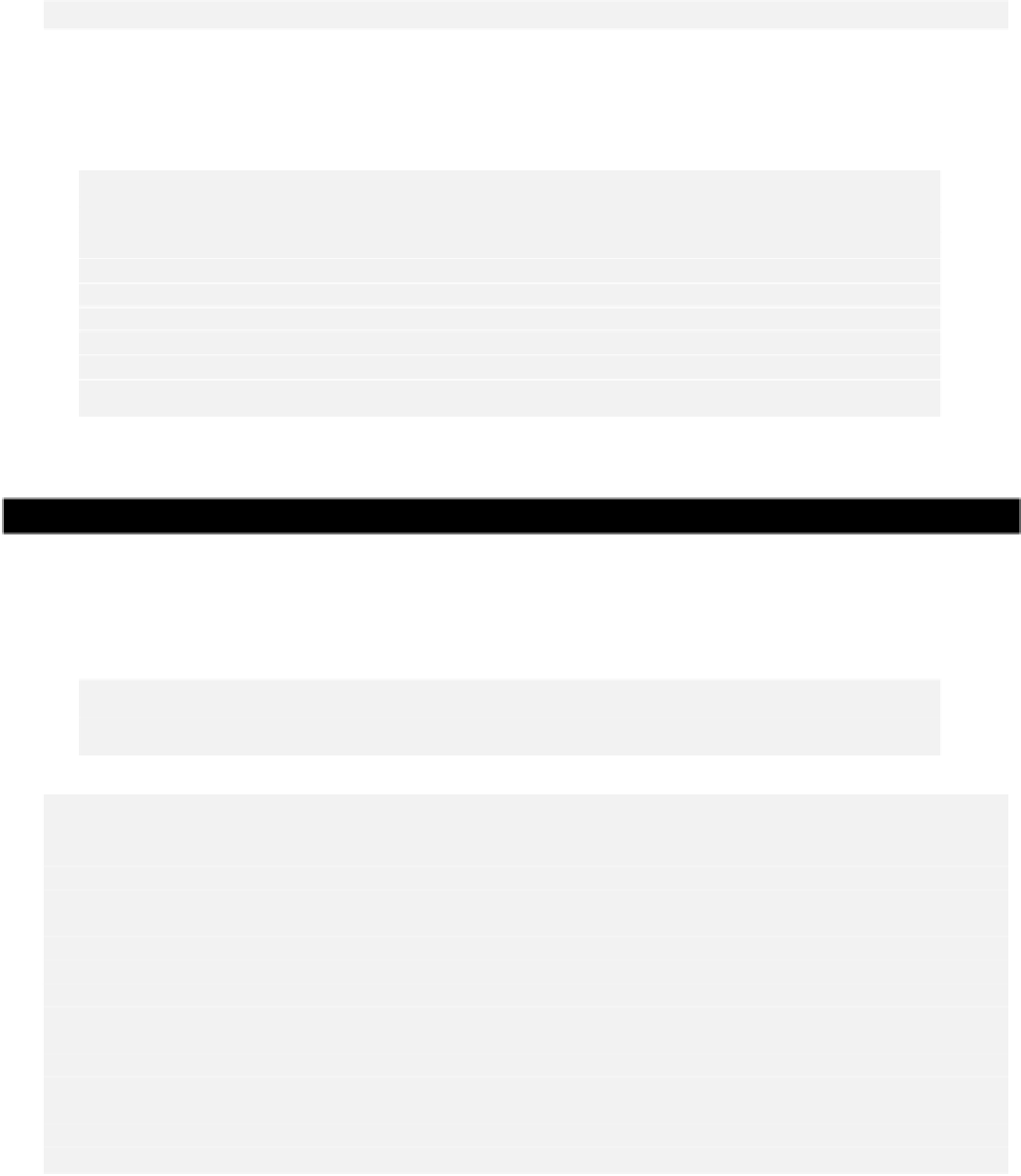













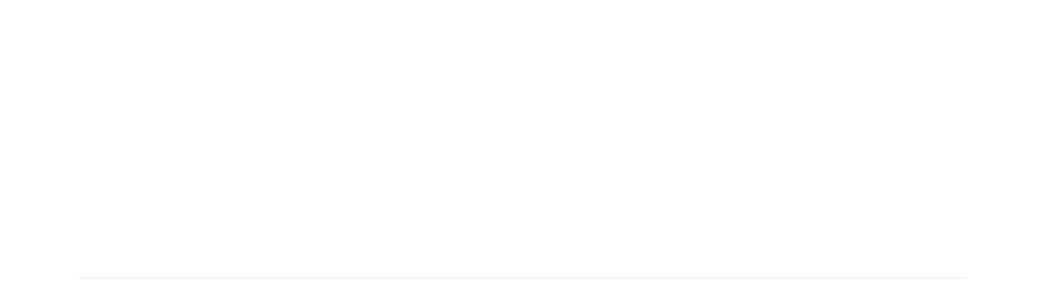