Java Reference
In-Depth Information
}
public double ouncesToGrams(double ounces) {
return ounces*OUNCE
_
TO
_
GRAM;
}
// Definition of the rest of the class...
}
You cannot create objects of type
MyClass
. To arrive at a useful class, you must define a subclass of
MyClass
that implements the remaining methods in the interface. The declaration of the class as
abstract
is mandatory when you don't implement all of the methods that are declared in an interface.
The compiler will complain if you forget to do this.
Now we know how to write the code to implement an interface, we can tie up something we met earlier
in this chapter. We mentioned that you need to implement the interface
Cloneable
to use the
inherited method
clone()
. In fact this interface is empty with no methods or constants, so all you need
to do to implement it in a class is to specify that the class in question implements. This means that you
just need to write something like:
public MyClass implements Cloneable {
// Detail of the class...
}
The sole purpose of the
Cloneable
interface is to act as a flag signalling that you are prepared to allow
objects of your class to be cloned. Even though you have defined a public
clone()
method in your
class, the compiler will not permit the
clone()
method to be called for objects of your class type
unless you also specify that your class implements
Cloneable
.
Extending Interfaces
You can define one interface based on another by using the keyword
extends
to identify the base
interface name. This is essentially the same form as we use to derive one class from another. The
interface doing the extending acquires all the methods and constants from the interface it extends. For
example, the interface
Conversions
would perhaps be more useful if it contained the constants that
the interface
ConversionFactors
contains.
We could do this by defining the interface
Conversions
as:
public interface Conversions extends ConversionFactors {
double inchesToMillimeters (double inches);
double ouncesToGrams(double ounces);
double poundsToGrams(double pounds);
double hpToWatts(double hp);
double wattsToHP(double watts);
}
Now the interface
Conversions
also contains the members of the interface
ConversionFactors
.
Any class implementing the
Conversions
interface will have the constants from
ConversionFactors
available to implement the methods. Analogous to the idea of a superclass, the
interface
ConversionFactors
is referred to as a
super-interface
of the interface
Conversions
.
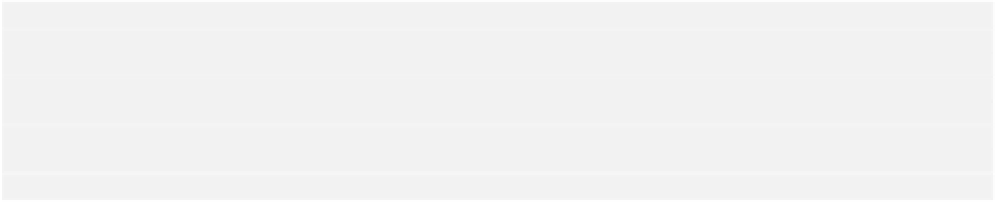








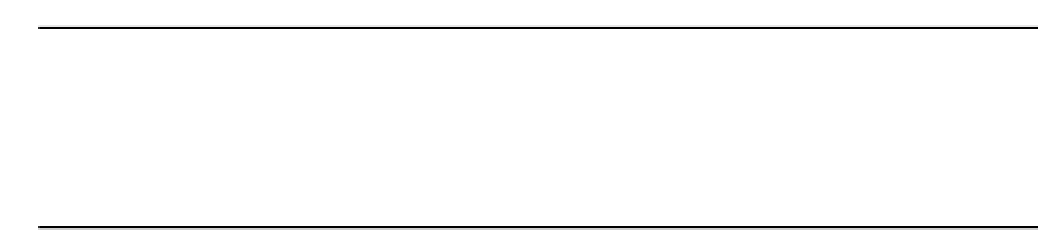