Java Reference
In-Depth Information
Although you cannot cast between unrelated objects, from
Spaniel
to
Duck
for instance, you can
achieve a conversion by writing a suitable constructor but obviously, only where it makes sense to do
so. You just write a constructor in the class to which you want to convert, and make it accept an object
of the class you are converting from as an argument. If you really thought
Spaniel
to
Duck
was a
reasonable conversion, you could add the constructor to the
Duck
class:
public Duck(Spaniel aSpaniel) {
// Back legs off, and staple on a beak of your choice...
super("Duck"); // Call the base constructor
name = aSpaniel.getName();
breed = "Barking Coot"; // Set the duck breed for a converted Spaniel
}
This assumes you have added a method,
getName()
, in the class
Dog
which will be inherited in the
class
Spaniel
, and which returns the value of
name
for an object. This constructor accepts a
Spaniel
and turns out a
Duck
. This is quite different from a cast though. This creates a completely new object
that is separate from the original, whereas a cast presents the same object as a different type.
When to Cast Objects
You will have cause to cast objects in both directions through a class hierarchy. For example, whenever
you execute methods polymorphically, you will be storing objects in a variable of a base class type, and
calling methods in a derived class. This will generally involve casting the derived class objects to the
base class. Another reason you might want to cast up through a hierarchy is to pass an object of several
possible subclasses to a method. By specifying a parameter as base class type, you have the flexibility to
pass an object of any derived class to it. You could pass a
Dog
,
Duck
or
Cat
to a method as an
argument for a parameter of type
Animal
, for instance.
The reason you might want to cast down through a class hierarchy is to execute a method unique to a
particular class. If the
Duck
class has a method
layEgg(),
for example, you can't call this using a
variable of type
Animal
, even though it references a
Duck
object. As we have already said, casting
downwards through a class hierarchy always requires an explicit cast.
Try It Out - Casting Down to Lay an Egg
We'll amend the
Duck
class and use it along with the
Animal
class in an example. Add
layEgg()
to
the
Duck
class as:
public class Duck extends Animal {
public void layEgg() {
System.out.println("Egg laid");
}
// Rest of the class as before...
}
If you now try to use this with the code:
public class LayEggs {
public static void main(String[] args) {

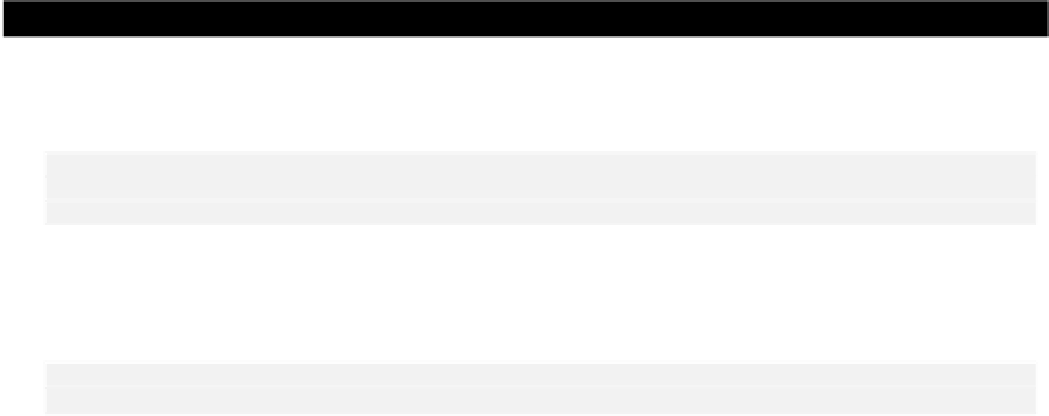












