Java Reference
In-Depth Information
// Return a String full of a cat's details
public String toString() {
return super.toString() + "\nIt's " + name + " the " + breed;
}
// A miaowing method
public void sound() {
System.out.println("Miiaooww");
}
private String name; // Name of a cat
private String breed; // Cat breed
}
Just to make it a crowd, we can derive another class - of ducks:
public class Duck extends Animal {
public Duck(String aName) {
super("Duck"); // Call the base constructor
name = aName; // Supplied name
breed = "Unknown"; // Default breed value
}
public Duck(String aName, String aBreed) {
super("Duck"); // Call the base constructor
name = aName; // Supplied name
breed = aBreed; // Supplied breed
}
// Return a String full of a duck's details
public String toString() {
return super.toString() + "\nIt's " + name + " the " + breed;
}
// A quacking method
public void sound() {
System.out.println("Quack quackquack");
}
private String name; // Duck name
private String breed; // Duck breed
}
You can fill the whole farmyard, if you need the practice, but three kinds of animal are sufficient to
show you how polymorphism works.
We need to make one change to the class
Animal
. To select the method
sound()
dynamically for
derived class objects, it needs to be a member of the base class. We can add a content-free version of
sound()
to the class
Animal
:
class Animal {







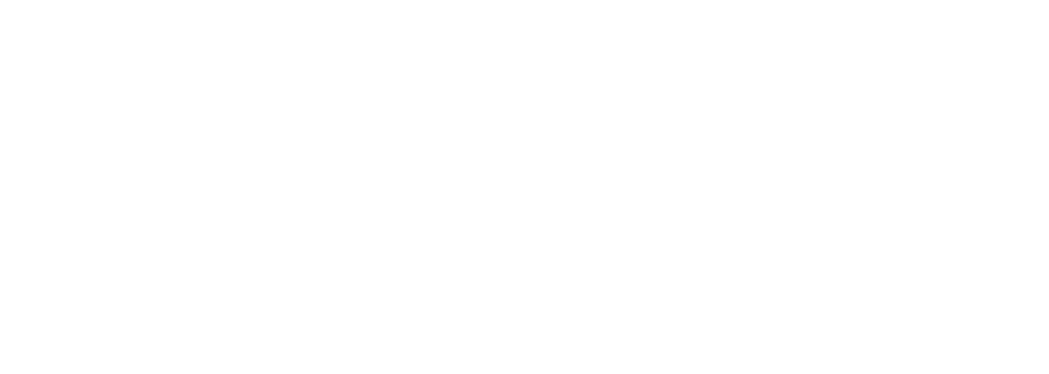