Java Reference
In-Depth Information
Since each pair of
Point
objects will define a
Line
object, we need one less element in the array
lines
than we have in the
points
array. We create the elements of the lines array in the second
for
loop using
successive
Point
objects, and accumulate the total length of all the line segments by adding the length of
each
Line
object to
totalLength
as it is created. On each iteration of the
for
loop, we output the details
of the current line. Finally, we output the value of
totalLength
, which in this case is 45.
Note that the
import
statement adds the classes from the package
Geometry
to our program. These
classes can be added to any application using the same
import
statement. You might like to try putting
the classes in the
Geometry
package in a JAR file and try it out as an extension. Let's look at one other
aspect of generating your own packages - compiling just the classes in the package without any program
that makes use of them. We can demonstrate how this can be done on our
Geometry
package if you
delete the
Line.class
and
Point.class
files from the package directory.
First make the directory,
C:\TryPackage
, that contains the package directory, current. Now you can
compile just the classes in the
Geometry
package with the command:
javac -classpath "C:\TryPackage" Geometry/*.java
This will compile both the
Line
and
Point
classes so you should see the
.class
files restored in the
Geometry
directory. The files to be compiled are specified relative to the current directory as
Geometry/*.java.
Under Microsoft Windows this could equally well be
Geometry\*.java
. This
specifies all files in the
Geometry
subdirectory to the current directory. The classpath must contain the
path to the package directory, otherwise the compiler will not be able to find the package. We have
defined it here using the
-classpath
option. We have not specified the current directory in classpath
since we do not have any files there that need to be compiled. If we had included it in classpath it would
not have made any difference - the classes in the
Geometry
package would compile just the same.
Nested Classes
All the classes we have defined so far have been separate from each other - each stored away in its own
file. Not all classes have to be defined like this. You can put the definition of one class inside the
definition of another class. The inside class is called a
nested
class
. A nested class can itself have another
class nested inside it, if need be.
When you define a nested class, it is a member of the enclosing class in much the same way as other
members. A nested class can have an access attribute just like other class members, and the accessibility
from outside the enclosing class is determined by the attributes in the same way.
public class Outside {
// Nested class
public class Inside {
// Details of Inside class...
}
// More members of Outside class...
}
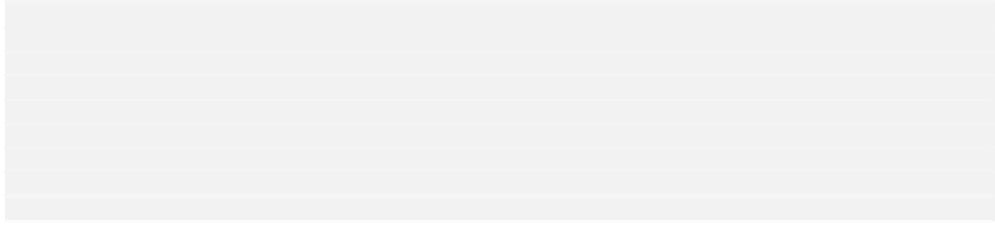





