Java Reference
In-Depth Information
How It Works
The method
power()
has two parameters, the value
x
and the power
n
. The method performs four
different actions, depending on the value of
n
:
n
>1
A recursive call to
power()
is made with
n
reduced by 1, and the value returned is
multiplied by
x
. This is effectively calculating x
n
as x times x
n-1
.
n
<0
x
-n
is equivalent to
1
/xn
so this is the expression for the return value. This involves a
recursive call to
power()
with the sign of
n
reversed.
n
=0
x
0
is defined as 1, so this is the value returned.
n
=1
x
1
is
x
, so
x
is returned.
Just to make sure the process is clear we can work through the sequence of events as they occur in the
calculation of 5
4
.
Level
Description
Relevant Code
1
The first call of the
power()
method passes 5.0 and 4 as
arguments. Since the second
argument,
n
, is greater than 1, the
power()
method is called again in
the
return
statement, with the
second argument reduced by 1.
Power(5.0, 4) {
if(n > 1)
return 5.0*power(5.0, 4-1);
...
}
2
The second call of the
power()
method passes 5.0 and 3 as
arguments. Since the second
argument, n, is still greater than 1,
the
power()
method is called again
in the
return
statement with the
second argument reduced by 1.
Power(5.0, 3) {
if(n > 1)
return 5.0*power(5.0, 3-1);
...
}
Power(5.0, 2) {
if(n > 1)
return 5.0*power(5.0, 2-1);
...
}
3
The third call of the
power()
method passes 5.0 and 2 as
arguments. Since the second
argument,
n
, is still greater than 1,
the
power()
method is called again,
with the second argument again
reduced by 1.
4
The fourth call of the
power()
method passes 5.0 and 1 as
arguments. Since the second
argument,
n
, is not greater than 1,
the value of the first argument, 5.0,
is returned to level
3
.
Power(5.0, 1) {
if(n > 1)
...
else
return 5.0;
}





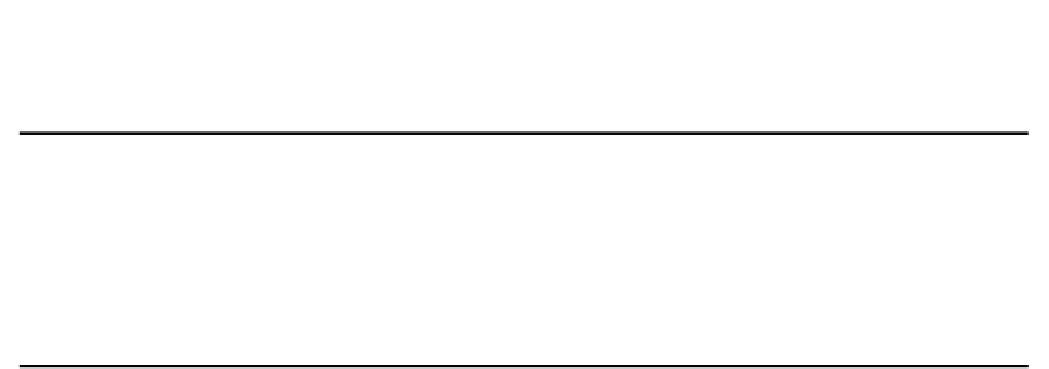