Java Reference
In-Depth Information
This is illustrated in the following diagram.
public static void main(String[] args){
...
x = obj.mean( 3.0 , 5.0 );
...
This value
is used for
value2
}
This value
is used for
value1
This value substitutes
for the method name
where it was called
This variable only
exists while the
method is executing
double mean( double value1 , double value2 ){
double result = ( value1 + value2 )/ 2.0;
return result;
}
This is the value
returned by the
method
Here we have the definition of a method
mean()
. This can only appear within the definition of a class,
but the rest of the class definition has been omitted so as not to clutter up the diagram. You can see that
the method has two parameters,
value1
, and
value2
, both of which are of type
double
, that are used
to refer to the arguments
3.0
and
5.0
respectively within the body of the method. Since this method
has not been defined as
static
, you can only call it for an object of the class. We call
mean()
in our
example for the object,
obj
.
When you call the method from another method (from
main()
in this case, but it could be from some
other method), the values of the arguments passed are the initial values assigned to the corresponding
parameters. You can use any expression you like for an argument when you call a method, as long as
the value it produces is of the same type as the corresponding parameter in the definition of the method.
With our method
mean()
, both parameters are of type
double
, so both argument values must always
be of type
double
.
The method
mean()
declares the variable
result
, which only exists within the body of the method.
The variable is created each time you execute the method and it is destroyed when execution of the
method ends. All the variables that you declare within the body of a method are local to the method,
and are only around while the method is being executed. Variables declared within a method are called
local
variables
because they are local to the method. The scope of a local variable is as we discussed in
Chapter 2, and local variables are not initialized automatically. If you want your local variables to have
initial values you must supply the initial value when you declare them.
How Argument Values Are Passed to a Method
You need to be clear about how your argument values are passed to a method, otherwise you may run
into problems. In Java, all argument values that belong to one of the basic types are transferred to a
method using what is called the
pass-by-value
mechanism. How this works is illustrated below.

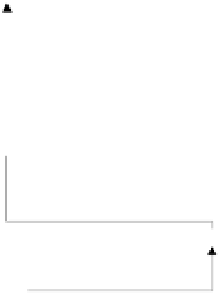
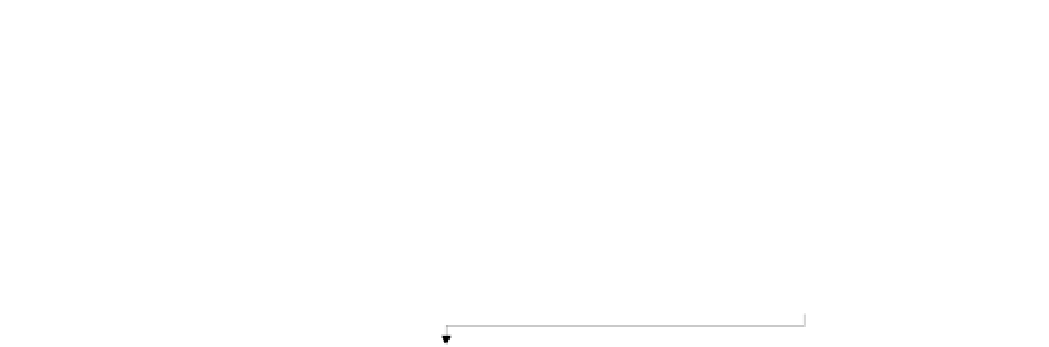