Java Reference
In-Depth Information
String Interning
Having convinced you of the necessity for using the
equals
method for comparing strings, we can now
reveal that there is a way to make comparing strings with the
==
operator effective. The mechanism to
make this possible is called
string interning
. String interning ensures that no two
String
objects
encapsulate the same string so all
String
objects encapsulates unique strings. This means that if two
String
variables reference strings that are identical, the references must be identical too. To put it
another way, if two
String
variables contain references that are not equal, they must refer to strings
that are not equal. So how do we arrange that all
String
objects encapsulate unique strings? You just
call the
intern()
method for every new
String
object that you create. For instance, let's amend a bit
of an earlier example:
String string1 = "Too many ";
String string2 = "cooks";
String string3 = "Too many cooks";
// Make string1 and string3 refer to separate strings that are identical
string1 += string2;
string1 = string1.intern(); // Intern string1
The
intern()
method will check the string referenced by
string1
against all the
String
objects
currently in existence. If it already exists, the current object will be discarded and
string1
will contain
a reference to the existing object encapsulating the same string. As a result, the expression
string1
==
string3
will evaluate to
true
, whereas without the call to
intern()
it evaluated to
false
.
All string constants and constant
String
expressions are automatically interned. Thus if you add
another variable to the code fragment above:
String string4 = "Too " +"many ";
the reference stored in
string4
will be automatically the same as the reference stored in
string1
.
Only
String
expressions involving variables need to be interned. We could have written the statement
that created the combined string to be stored in
string1
with the statement:
string1 = (string1 + string2).intern();
This now interns the result of the expression
(string1
+
string2)
, ensuring that the reference
stored in
string1
will be unique.
String interning has two benefits. First, it reduces the amount of memory required for storing
String
objects
in your program. If your program generates a lot of duplicate strings then this will be significant. Second, it
allows the use of
==
instead of the
equals()
method when you want to compare strings for equality. Since
the
==
operator just compares two references, it will be much faster than the
equals()
method, which
involves a sequence of character by character comparisons. This implies that you
may
make your program
run much faster, but only in certain cases. Keep in mind that the
intern()
method has to use the
equals()
method to determine whether a string already exists. More than that, it will compare the current
string against a succession of, and possibly all, existing strings in order to determine whether the current
string is unique. Realistically you should stick to using the
equals()
method in the majority of situations
and only use interning when you are sure that the benefits outweigh the cost.

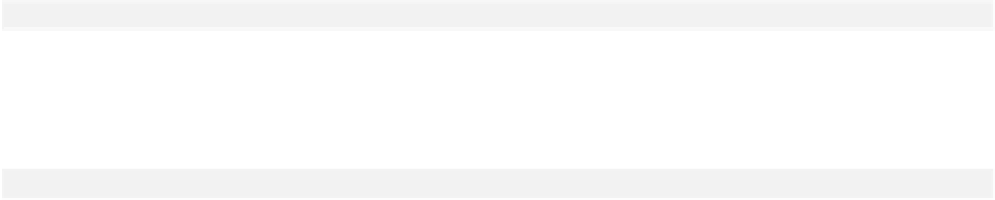










