Java Reference
In-Depth Information
String anyString = null; // String variable that doesn't reference a string
The actual value it stores in this situation is referred to as
null
, so you can test whether a
String
variable refers to anything or not by a statement such as:
if(anyString == null)
System.out.println("anyString does not refer to anything!");
The variable
anyString
will continue to be
null
until you use an assignment to make it reference a
particular string. Attempting to use a variable that has not been initialized is an error. When you declare
a
String
variable, or any other variable that is not an array, in a block of code without initializing it,
the compiler can detect any attempts to use the variable before it has a value assigned, and will flag it as
an error. As a rule, you should always initialize variables as you declare them.
Arrays of Strings
Since
String
variables are objects, you can create arrays of strings. You declare an array of
String
objects with the same mechanism that we used to declare arrays of elements for the basic types. You just
use the type
String
in the declaration. For example, to declare an array of five
String
objects, you
could use the statement:
String[] names = new String[5];
It should now be apparent that the argument to the method
main()
is an array of
String
objects.
We can try out arrays of strings with a small example.
Try It Out - Twinkle, Twinkle, Lucky Star
Let's create a console program to generate your lucky star for the day.
public class LuckyStars {
public static void main(String[] args) {
String[] stars = {
"Robert Redford" , "Marilyn Monroe",
"Boris Karloff" , "Lassie",
"Hopalong Cassidy", "Trigger"
};
System.out.println("Your lucky star for today is "
+ stars[(int)(stars.length*Math.random())]);
}
}
How It Works
This program creates the array
stars
, of type
String
. The array length will be set to however many
initializing values appear between the braces in the declaration statement, six in this case.
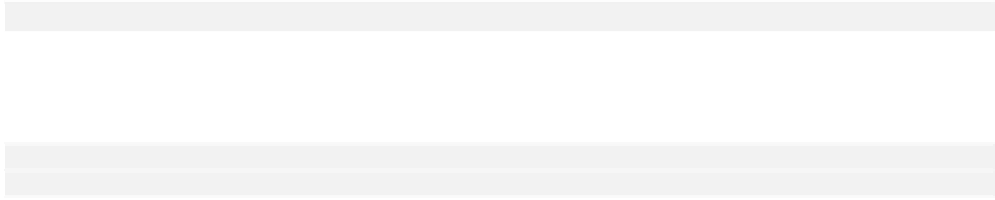
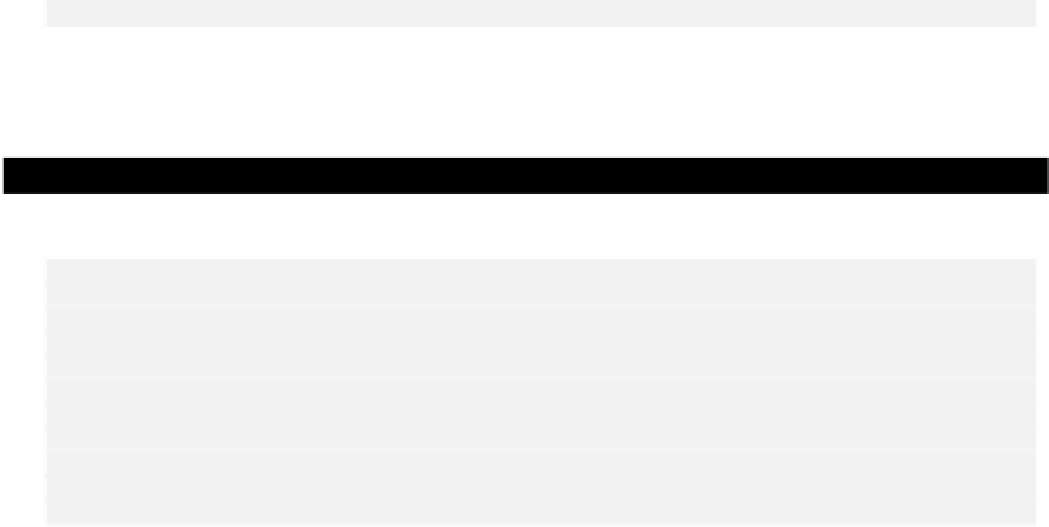











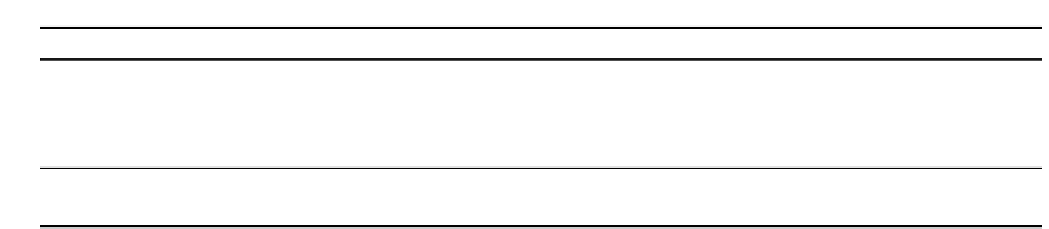